python获取list的形状
时间: 2024-10-14 18:15:22 浏览: 48
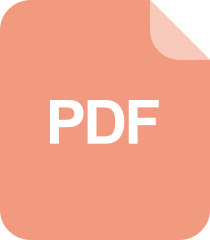
Python 元组(Tuple)操作详解

在Python中,`imgArray[x][y].shape[:2]` 是用来获取二维数组(如图像)的维度信息,这里的 `shape[:2]` 返回的是第一个两个元素,通常代表宽度和高度。对于列表 (`list`),`shape` 并不是一个标准概念,但我们可以理解为它们的长度,即元素的数量。
如果你有一个二维列表 `imgArray`,你可以通过 `len()` 函数来获取其形状(即元素数量),这类似于 `imgArray.shape` 的概念:
```python
# 假设我们有一个二维列表
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# 获取列表的形状(即元素数量)
list_shape = len(my_list)
print(f"List shape (number of rows): {list_shape}")
# 对于每一行,如果它是二维的(比如每个元素本身也是列表)
for row in imgArray:
if isinstance(row, list):
row_shape = len(row)
print(f"Row shape (number of elements): {row_shape}")
```
请注意,这里假设 `imgArray` 可能包含了非列表元素,所以我们在遍历时检查每一项是否仍为列表。
阅读全文
相关推荐
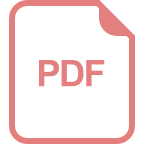



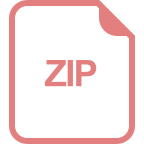



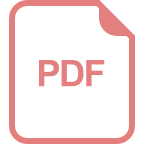







