C语言中怎么在字符串中找出重复的字符
时间: 2024-12-15 15:13:59 浏览: 3
在C语言中,要找出字符串中重复的字符,可以采用哈希表(如`char hash[256]`,因为ASCII码有256个字符)或者数组+遍历的方法。以下是两种基本思路:
**方法一:哈希表**
1. 初始化一个大小为256的布尔型数组,所有元素设为false。
2. 遍历字符串,对于每个字符,如果该字符对应的数组位置为false,则标记为true,并继续下一个字符。
3. 如果某个字符对应的数组位置为true,说明这个字符在字符串中有重复。
```c
#include <stdio.h>
int main() {
char str[] = "hello";
int hash[256] = {0};
for (int i = 0; str[i]; i++) {
if (hash[str[i]]) {
printf("字符 '%c' 重复\n", str[i]);
} else {
hash[str[i]] = 1;
}
}
return 0;
}
```
**方法二:数组+遍历**
1. 创建一个长度和输入字符串一样大的数组,初始化全为0。
2. 再次遍历字符串,每次遇到字符时,将对应索引位置的计数加1。
3. 检查数组,找到计数值大于1的字符即为重复字符。
```c
#include <stdio.h>
int count[128] = {0}; // ASCII字符集范围
void findDuplicates(char* str) {
for (int i = 0; str[i]; i++) {
count[str[i]]++;
}
for (int i = 0; i < 128; i++) {
if (count[i] > 1 && i >= 'a' && i <= 'z') {
printf("字符 '%c' 重复\n", i);
}
}
}
int main() {
char str[] = "hello";
findDuplicates(str);
return 0;
}
```
阅读全文
相关推荐
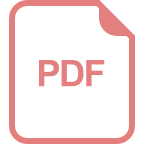
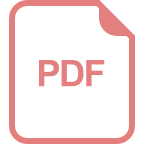
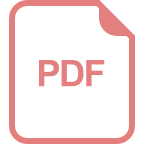
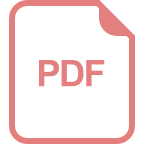
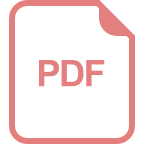
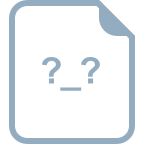
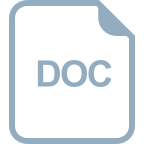
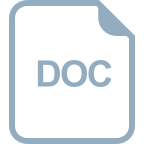
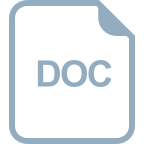
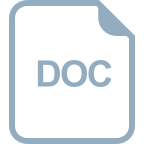
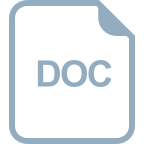
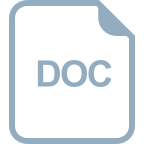
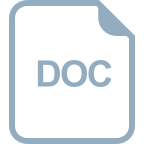





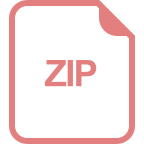
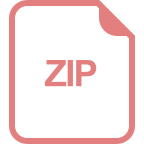