OpenCV C++实现车道线检测
时间: 2023-12-29 16:04:14 浏览: 72
OpenCV C++实现车道线检测的基本思路如下:
1. 读取图像并进行灰度化处理。
2. 对灰度图像进行高斯滤波,去除噪声。
3. 对滤波后的图像进行Canny边缘检测,得到边缘图像。
4. 对边缘图像进行霍夫变换,检测直线。
5. 对检测到的直线进行筛选和拟合,得到车道线。
下面是OpenCV C++实现车道线检测的代码示例:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像
Mat src = imread("test.jpg");
// 灰度化处理
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
// 高斯滤波
Mat blur;
GaussianBlur(gray, blur, Size(5, 5), 0);
// Canny边缘检测
Mat edges;
Canny(blur, edges, 50, 150);
// 霍夫变换检测直线
vector<Vec2f> lines;
HoughLines(edges, lines, 1, CV_PI / 180, 100);
// 筛选和拟合直线
vector<Vec4i> lanes;
for (size_t i = 0; i < lines.size(); i++)
{
float rho = lines[i][0], theta = lines[i][1];
if (theta < CV_PI / 4 || theta > 3 * CV_PI / 4)
{
Point pt1(rho / cos(theta), 0);
Point pt2((rho - edges.rows * sin(theta)) / cos(theta), edges.rows); lanes.push_back(Vec4i(pt1.x, pt1.y, pt2.x, pt2.y));
}
}
// 绘制车道线
Mat result = src.clone();
for (size_t i = 0; i < lanes.size(); i++)
{
line(result, Point(lanes[i][0], lanes[i][1]), Point(lanes[i][2], lanes[i][3]), Scalar(0, 0, 255), 3, LINE_AA);
}
// 显示结果
imshow("result", result);
waitKey(0);
return 0;
}
```
相关推荐
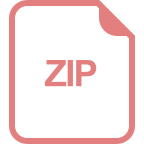
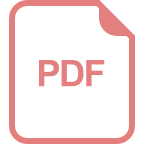











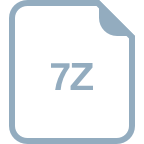
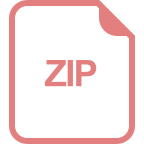
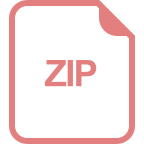
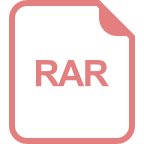