python爬虫万能代码
时间: 2023-11-08 13:02:08 浏览: 110
对于爬虫来说,并没有所谓的万能代码,因为每个网站的结构和反爬机制都不同。不过,下面是一个简单的爬取网页内容的示例代码:
```python
import requests
url = 'https://example.com' # 替换为你要爬取的网址
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print('请求失败')
```
这段代码使用了`requests`库发送HTTP请求,并获取网页的内容。你可以将其中的`'https://example.com'`替换为你要爬取的具体网址。
相关问题
python爬虫基本代码
Python爬虫是一种用于自动化获取互联网上数据的技术。下面是Python爬虫的基本代码结构:
1. 导入所需的库:
```python
import requests
from bs4 import BeautifulSoup
```
2. 发送HTTP请求并获取页面内容:
```python
url = "http://example.com" # 要爬取的网页URL
response = requests.get(url)
```
3. 解析页面内容:
```python
soup = BeautifulSoup(response.text, "html.parser")
```
4. 提取所需的数据:
```python
# 通过标签名提取数据
data = soup.find("tag_name").text
# 通过CSS选择器提取数据
data = soup.select("css_selector").text
# 通过XPath提取数据(需要安装lxml库)
data = soup.xpath("xpath_expression").text
```
5. 存储或处理数据:
```python
# 存储到文件
with open("data.txt", "w", encoding="utf-8") as file:
file.write(data)
# 处理数据
processed_data = process_data(data)
```
以上是Python爬虫的基本代码结构,你可以根据具体需求进行修改和扩展。
Python爬虫百度代码
Python 爬虫可以使用 requests 库来发送 HTTP 请求获取网页内容,然后使用 Beautiful Soup 库或 lxml 库等解析 HTML 页面内容,从而实现爬取百度搜索结果的功能。以下是一个简单的 Python 爬虫百度搜索结果的代码示例:
```python
import requests
from bs4 import BeautifulSoup
def baidu_search(keyword):
url = 'https://www.baidu.com/s?wd=' + keyword
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Edge/16.16299'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'lxml')
results = soup.find_all('div', class_='result')
for result in results:
title = result.find('a').text.strip()
link = result.find('a').get('href')
print(title + '\n' + link + '\n')
# 调用函数进行百度搜索
baidu_search('Python 爬虫')
```
阅读全文
相关推荐
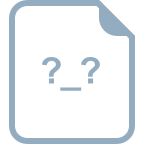
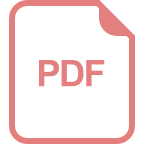
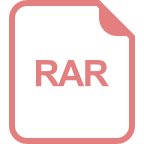













