在C#窗体中,已配置GDAL库,如何实现对picturebox控件中的遥感影像进行平移缩放的操作?
时间: 2024-10-24 15:05:49 浏览: 21
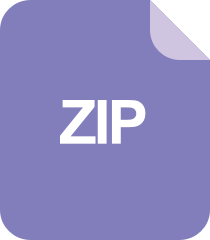
gdal.zip_c# gdal_gdal函数库_gdal库_遥感图像处理_遥感影像处理
在C# Windows Form中,若已配置了GDAL库(Geospatial Data Abstraction Library),你可以利用GDAL的功能来操作PictureBox控件中的遥感图像。首先,你需要读取图片到一个GDAL可以处理的格式,比如BMP、TIFF或GeoTIFF。然后,可以使用`IDataset`或`IGDALRasterBand`等接口来处理图像数据。
以下是简单的步骤:
1. **加载遥感影像**:
使用`GDAL.Open`方法打开文件,并将其转换为`IDataset`或`IRasterBand`对象,例如:
```csharp
private IGDALRasterBand rasterBand;
private IDataset dataset;
// 加载imagefile
dataset = Gdal.Open("path_to_your_raster_file", Access.GA_ReadOnly);
if (dataset != null)
{
rasterBand = dataset.GetRasterBand(1); // 获取第一层
}
```
2. **获取PictureBox的Graphics对象**:
```csharp
Graphics g = pictureBox1.CreateGraphics();
```
3. **图像操作(如缩放和平移)**:
- **缩放**:使用`g.DrawImage`方法,传入缩放后的图像和位置:
```csharp
int newX, newY;
// 缩放比例因子
double scale = 0.5;
newX = pictureBox1.Width / scale;
newY = pictureBox1.Height / scale;
g.DrawImage(rasterBand.ReadAsBitmap(new Rectangle(0, 0, newX, newY)), 0, 0);
```
- **平移**:调整pictureBox的位置即可,不需要GDAL直接支持,因为它是UI元素,可以独立移动:
```csharp
pictureBox1.Location = new Point(pictureBox1.Location.X + xShift, pictureBox1.Location.Y + yShift);
```
4. **释放资源**:
当不再需要时,记得关闭`dataset`和`rasterBand`的引用以及GDAL文件:
```csharp
if (rasterBand != null)
{
rasterBand.Dispose();
}
if (dataset != null)
{
dataset.Dispose();
}
```
阅读全文
相关推荐
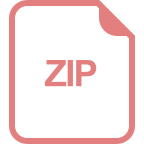
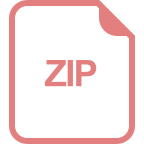

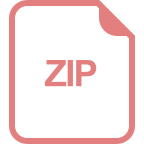
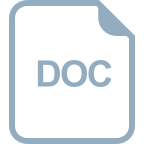
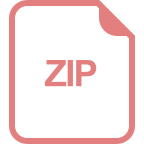
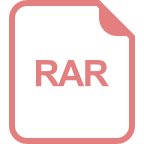
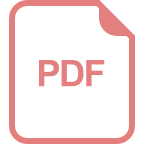
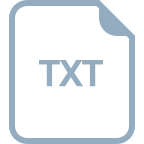
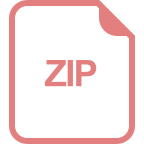
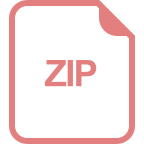
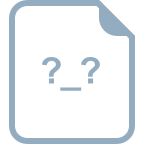
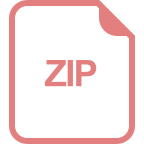
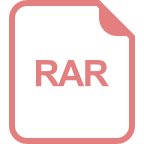
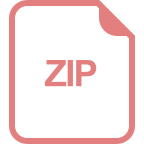
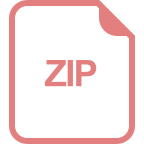
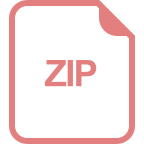