在C#窗体中,已配置GDAL库,如何实现将form1中picturebox控件中的遥感影像传递到form2中的picturebox控件中?
时间: 2024-10-24 09:05:47 浏览: 23
在C#中,如果你已经配置了GDAL库(GDAL是一个开源地理空间数据访问库),你可以通过以下几个步骤将Form1中的PictureBox控件中的遥感影像数据传递到Form2:
1. **加载遥感影像**:首先,在Form1中读取并加载遥感影像文件。这通常涉及到创建一个`Bitmap`对象或`Image`对象,然后设置PictureBox的图片属性。例如:
```csharp
using System.Drawing;
// ...
private void LoadImageToPictureBox(Bitmap image)
{
pictureBox1.Image = image;
}
// 然后在需要的地方加载遥感影像
string imagePath = "path_to_your_raster_image";
Bitmap rasterImage = new Bitmap(imagePath);
LoadImageToPictureBox(rasterImage);
```
2. **保存图像数据**:GDAL库可能会提供将图像转换成字节流或其他适合在网络间传输的数据格式的方法。这里假设有一个`SaveAsBytes`函数,它能将`Bitmap`转换成字节数组:
```csharp
byte[] imageData;
if (gdalLibraryInstance != null) // gdalLibraryInstance是你之前配置好的GDAL实例
{
imageData = gdalLibraryInstance.SaveAsBytes(rasterImage);
}
else
{
throw new Exception("GDAL library not initialized properly.");
}
```
3. **在Form2中接收和显示图像**:在Form2的构造函数或适当的地方,可以添加一个接受字节数组并重新创建`Bitmap`的方法:
```csharp
private void Form2_Load(object sender, EventArgs e)
{
if (imageData != null)
{
Bitmap receivedImage = BitmapFactory.FromByteArray(imageData);
pictureBox2.Image = receivedImage;
}
}
```
4. **传递数据**:在Form1关闭、销毁或你想发送图像的时候,将`imageData`作为参数传给Form2的某个方法。
注意:上述代码并未涵盖所有细节,实际实现可能需要处理异常,并确保GDAL库的引用路径和初始化正确。
阅读全文
相关推荐
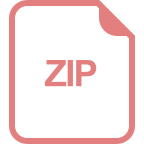
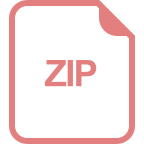
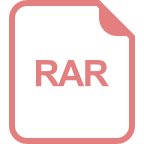

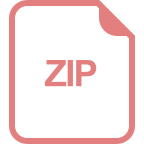
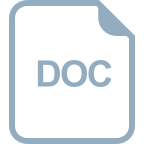
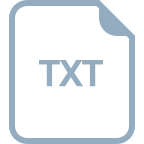
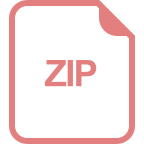
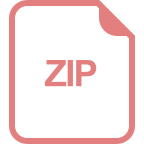
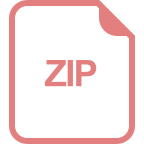
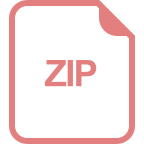
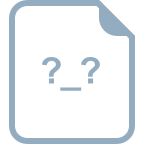
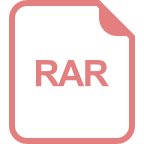
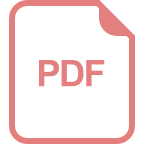
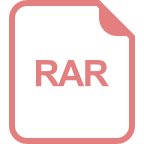
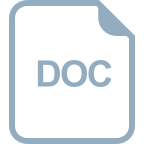
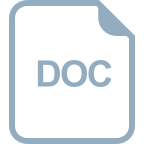
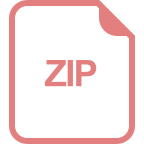
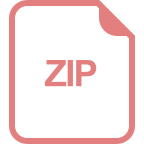