用python读取大量excel数据,根据每行数据post多线程请求后,返回的内容添加一列
时间: 2024-10-27 20:11:35 浏览: 23
在Python中,可以使用pandas库读取Excel文件,并结合`concurrent.futures`模块实现多线程并发处理。首先,你需要安装`pandas`和`openpyxl`库,如果你还没有安装,可以使用下面的命令:
```bash
pip install pandas openpyxl
```
然后,你可以按照以下步骤操作:
1. **读取Excel数据**:
使用`pandas`的`read_excel`函数加载Excel文件。
```python
import pandas as pd
# 加载Excel文件
df = pd.read_excel('your_file.xlsx')
```
2. **准备数据处理函数**:
创建一个函数,该函数接受一行数据,发送POST请求并获取响应结果。
```python
import requests
from threading import Thread
def process_row(row_data):
url = 'http://example.com/api' # 替换为你实际的API地址
response = requests.post(url, json=row_data)
# 将响应内容转换为你需要的一列数据并添加到row_data字典中
row_data['response_column'] = response.json() # 假设响应是一个json
return row_data
```
3. **多线程遍历并处理数据**:
使用`ThreadPoolExecutor`创建一个线程池来并发处理每一行数据。
```python
from concurrent.futures import ThreadPoolExecutor
# 确保数据框有index,便于后续操作
df = df.set_index('index')
with ThreadPoolExecutor(max_workers=5) as executor: # 根据需求调整最大工作线程数
results = {i: executor.submit(process_row, df.loc[i]) for i in df.index}
# 当所有任务完成,收集结果
data_with_response = {}
for index, future in results.items():
data_with_response[index] = future.result()
```
4. **合并原始数据和新列**:
最后,将处理后的数据更新回DataFrame中。
```python
df_processed = pd.DataFrame(data_with_response).reset_index()
df = df.merge(df_processed, on='index', how='left') # 或者根据实际需要选择其他合并方式
```
阅读全文
相关推荐
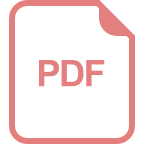
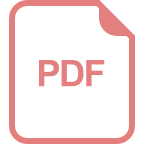
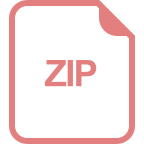
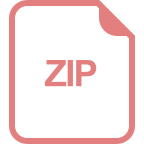
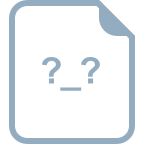
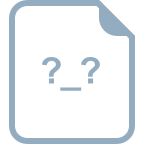
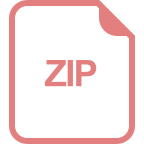
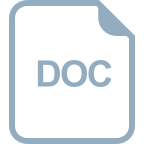
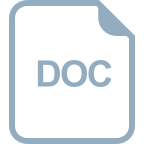
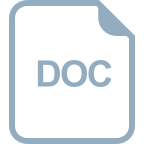
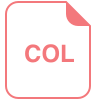
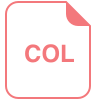
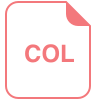
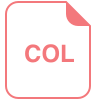
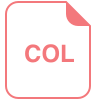
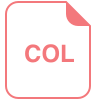
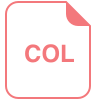
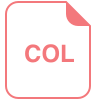
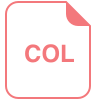