用C++创建一个文件,用面向文件的输入输出流增删文件内容,合并使用标准流对象进行输入输出。代码简单易懂
时间: 2024-10-23 21:20:28 浏览: 23
在C++中,你可以使用`fstream`库来进行文件操作,包括读取、写入以及合并。下面是一个简单的示例,展示了如何打开一个文件,添加新的内容,以及读取文件:
```cpp
#include <iostream>
#include <fstream>
#include <string>
// 定义函数用于向文件追加内容
void appendToFile(const std::string& filename, const std::string& content) {
std::ofstream outfile(filename, std::ios::app); // 'app'表示追加模式
if (outfile.is_open()) {
outfile << content << "\n"; // 在每一行末尾添加换行符
outfile.close();
std::cout << "Content appended to file successfully.\n";
} else {
std::cerr << "Failed to open the file for appending.\n";
}
}
// 定义函数用于从文件读取内容并输出到控制台
void readFromFile(const std::string& filename) {
std::ifstream infile(filename);
if (infile.is_open()) {
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl; // 输出文件内容到控制台
}
infile.close();
} else {
std::cerr << "Failed to open the file for reading.\n";
}
}
int main() {
// 向文件追加内容
appendToFile("example.txt", "Hello from C++!");
// 读取并打印文件内容
readFromFile("example.txt");
return 0;
}
```
在这个例子中,我们首先创建了两个函数:`appendToFile`用于向文件追加文本,`readFromFile`用于从文件读取并显示内容。然后在`main`函数中,我们先追加一些文本,接着立即读取并打印出来。
阅读全文
相关推荐
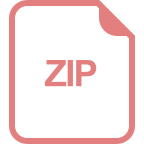
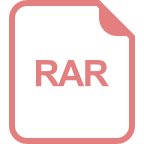
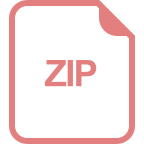















