用C语言实现循环队列
时间: 2024-12-22 15:22:30 浏览: 19
在C语言中,我们可以使用数组来实现一个简单的循环队列。循环队列的特点在于当队列满时,新的元素会从队列头部开始插入,而不会溢出;当队列空时,试图从队列头部取元素时会形成循环。下面是一个基本的循环队列结构体定义和相关的操作函数:
```c
#include <stdio.h>
#define MAX_SIZE 10
typedef struct {
int *queue; // 存储数据的数组
int front; // 队头指针
int rear; // 队尾指针
} Queue;
Queue* create_queue() {
Queue *q = (Queue*)malloc(sizeof(Queue));
if (!q) return NULL;
q->queue = (int*)malloc(MAX_SIZE * sizeof(int));
if (!q->queue) {
free(q);
return NULL;
}
q->front = -1;
q->rear = -1;
return q;
}
void enqueue(Queue *q, int data) {
if ((q->rear + 1) % MAX_SIZE == q->front) { // 判断队列是否已满
printf("Queue is full.\n");
return;
}
q->rear = (q->rear + 1) % MAX_SIZE; // 循环更新队尾
q->queue[q->rear] = data;
}
int dequeue(Queue *q) {
if (q->front == -1) { // 判断队列是否为空
printf("Queue is empty.\n");
return -1;
}
int data = q->queue[q->front]; // 读取并移除队头元素
q->front = (q->front + 1) % MAX_SIZE;
return data;
}
// 其他辅助函数,如检查队列是否为空、队列大小等
```
阅读全文
相关推荐









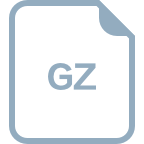



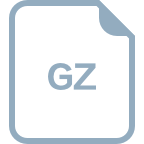


