from collections import deque #deque为双向队列,可以从队头尾快速操作 def Duplicate_check(s): queue = deque() #生成一个名字为queue的队列 for i in range(len(s)): c = s[i] # 队列中存在了当前字母,那么就不能再添加进去了,则跳过下列步骤,继续循环 if c in queue: continue # 如果队列中有元素,且队尾元素比当前字母大,且后面还会出现该队尾元素,那就弹出它。 while queue and queue[-1] > c and s.find(queue[-1], i) > 0: queue.pop() # 当前字母入队列 queue.append(c) res = "" # 将队列中的元素从队头至队尾弹出,就是答案了 while queue: res += queue.popleft() return res #返回答案 s = input() #输入 s = Duplicate_check(s) #调用函数处理 print(s) #输出 为这个程序做一个窗口化
时间: 2024-04-16 07:26:16 浏览: 14
要将这个程序窗口化,你可以使用Python的内库`tkinter`来创建一个简单的图形用户界面(GUI)。下面是一个窗口化版本的程序示例:
```python
from collections import deque
import tkinter as tk
def duplicate_check():
s = entry.get() # 获取输入框中的字符串
queue = deque()
for i in range(len(s)):
c = s[i]
if c in queue:
continue
while queue and queue[-1] > c and s.find(queue[-1], i) > 0:
queue.pop()
queue.append(c)
res = ""
while queue:
res += queue.popleft()
result_label.config(text=res) # 显示结果在标签中
# 创建GUI窗口
window = tk.Tk()
window.title("Duplicate Check")
window.geometry("300x150")
# 创建输入框
entry = tk.Entry(window)
entry.pack(pady=10)
# 创建按钮
button = tk.Button(window, text="Check", command=duplicate_check)
button.pack()
# 创建结果标签
result_label = tk.Label(window, text="")
result_label.pack(pady=10)
# 运行窗口主循环
window.mainloop()
```
运行以上代码,你将会看到一个具有输入框、按钮和结果标签的窗口。输入你要检查重复字符的字符串,点击“Check”按钮,程序将在结果标签中显示去重后的字符串。
相关推荐
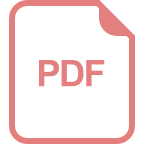
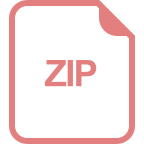
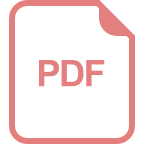















