C# 实现对字符串内容的 16位CRC校验码计算
时间: 2023-11-21 07:06:03 浏览: 46
你可以使用以下代码来实现对字符串内容的 16位CRC校验码计算:
```csharp
using System;
using System.Text;
public class CRC16
{
private ushort[] table;
public CRC16()
{
const ushort poly = 0xA001;
table = new ushort[256];
for (ushort i = 0; i < 256; i++)
{
ushort crc = 0;
for (int j = 0; j < 8; j++)
{
if (((crc ^ i) & 1) == 1)
{
crc = (ushort)((crc >> 1) ^ poly);
}
else
{
crc >>= 1;
}
i >>= 1;
}
table[i] = crc;
}
}
public ushort CalculateCRC(string input)
{
byte[] bytes = Encoding.ASCII.GetBytes(input);
ushort crc = ushort.MaxValue;
for (int i = 0; i < bytes.Length; i++)
{
byte index = (byte)(crc ^ bytes[i]);
crc = (ushort)((crc >> 8) ^ table[index]);
}
return crc;
}
}
```
你可以使用以下方式来调用这个类:
```csharp
string input = "Hello, world!";
CRC16 crc16 = new CRC16();
ushort crc = crc16.CalculateCRC(input);
Console.WriteLine("CRC16: 0x{0:X4}", crc);
```
这将输出字符串 "Hello, world!" 的16位CRC校验码。请注意,这里使用了C#内置的ASCII编码,如果你的字符串包含非ASCII字符,需要根据实际情况进行编码转换。
相关推荐
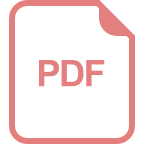














