如何爬取微博评论数据并使数据可视化
时间: 2023-09-07 12:11:55 浏览: 73
爬取微博评论数据并使数据可视化可以按照以下步骤进行:
1. 确定要爬取的微博,获取微博的URL。
2. 使用Python的requests库和正则表达式或BeautifulSoup库获取微博页面的HTML源代码。
3. 从HTML源代码中抓取评论数据,可以使用正则表达式或BeautifulSoup库。
4. 将评论数据存储到本地文件或数据库中,可以使用Python的pickle库、csv库或sqlite3库。
5. 使用Python的数据可视化库(如matplotlib、seaborn或Plotly)将数据可视化。
下面是一个简单的Python程序,可以爬取微博评论并将数据存储到本地csv文件中:
```python
import requests
from bs4 import BeautifulSoup
import csv
# 微博URL
url = 'https://weibo.com/xxx/yyy'
# 发送请求获取HTML源代码
response = requests.get(url)
html = response.text
# 使用BeautifulSoup解析HTML源代码
soup = BeautifulSoup(html, 'html.parser')
# 获取评论列表
comment_list = soup.find_all('div', class_='comment')
# 将评论数据存储到csv文件中
with open('comments.csv', 'w', encoding='utf-8', newline='') as f:
writer = csv.writer(f)
writer.writerow(['评论时间', '评论人', '评论内容'])
for comment in comment_list:
time = comment.find('span', class_='time').text
user = comment.find('a', class_='name').text
content = comment.find('div', class_='txt').text.strip()
writer.writerow([time, user, content])
```
接下来,可以使用Python的数据可视化库,如matplotlib,将评论数据可视化:
```python
import matplotlib.pyplot as plt
import pandas as pd
# 读取csv文件并转换为DataFrame对象
df = pd.read_csv('comments.csv', encoding='utf-8')
# 统计评论人出现次数
user_count = df['评论人'].value_counts()
# 绘制柱状图
plt.bar(user_count.index, user_count.values)
plt.xticks(rotation=90)
plt.xlabel('评论人')
plt.ylabel('评论数')
plt.show()
```
这段代码将评论人出现的次数绘制成柱状图,并展示在屏幕上。
相关推荐
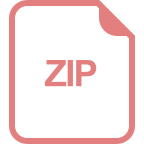
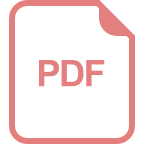














