python爬取微博评论数据可视化
时间: 2023-10-31 20:06:13 浏览: 156
Python爬取微博评论数据可视化可以通过以下步骤实现:
1. 使用Python的第三方库(如Selenium、BeautifulSoup等)模拟登录微博并获取目标用户的微博UID。
2. 使用微博API或者爬虫框架(如Scrapy)爬取目标用户的微博评论数据,并将数据保存到本地。
3. 使用Python的数据可视化库(如Matplotlib、Seaborn等)对评论数据进行可视化处理,例如绘制评论数随时间的变化趋势图、评论数最多的用户词云图等。
4. 可以使用Jupyter Notebook等工具将数据处理和可视化结果整合在一起,方便展示和分享。
相关问题
python爬取微博评论数据并可视化分析
非常感谢你的提问!
要爬取微博的评论数据,可以使用 Python 中的第三方库来实现,比如 BeautifulSoup 和 Requests。具体的流程大致如下:
1. 打开微博网页并模拟登录,获得 cookies。
2. 模拟浏览器操作,获取评论数据。
3. 解析评论数据并保存到本地文件或数据库中。
4. 使用 Matplotlib 或者其他可视化工具对数据进行可视化分析。
对于详细的代码实现和具体的流程细节,你可以在我的博客中查找相关文章进行学习:https://blog.csdn.net/ChitChat/article/details/119167532。
另外,如果你需要其他方面的帮助或者我理解错了你的问题,请随时告诉我。
python爬取微博评论数据并可视化分析代码
为了爬取微博评论数据,我们可以使用Python中的一个非常流行的网络爬虫框架——Scrapy。此外,我们还需要使用Selenium模拟用户登陆微博,并通过Selenium的WebDriver来控制浏览器进行数据爬取操作。以下是一个简单的示例代码,供参考:
```
import scrapy
from scrapy import Request
import time
import re
from selenium import webdriver
class WeiboSpider(scrapy.Spider):
name = 'weibo_comment'
allowed_domains = ['weibo.com']
def __init__(self):
self.chrome_options = webdriver.ChromeOptions()
self.chrome_options.add_argument('--headless')
self.chrome_options.add_argument('--disable-gpu')
self.browser = webdriver.Chrome(chrome_options=self.chrome_options)
def start_requests(self):
# 模拟登陆
self.browser.get('https://passport.weibo.com/visitor/visitor?entry=miniblog&a=enter&url=https://weibo.com/')
time.sleep(10)
self.browser.execute_script('document.getElementById("loginname").value="your_username";document.getElementById("password").value="your_password";')
self.browser.find_element_by_xpath('//div[@class="info_list login_btn"]/a[@class="W_btn_a btn_32px"]')
time.sleep(3)
# 获取评论数据
comment_url = 'https://weibo.com/ajax/statuses/repostTimeline?is_comment_base=1&id={}&page={}'
for i in range(1, 101):
url = comment_url.format('your_weibo_id', i)
yield Request(url=url, callback=self.parse)
def parse(self, response):
# 处理评论数据
html = response.text
pids = re.findall('"id":"(\d+)"', html)
cids = re.findall('"cid":"(\d+)"', html)
comments = re.findall('"text":"(.*?)".*?"created_at":"(.*?)".*?"user":{.*?:"(.*?)".*?}', html, re.S)
for i in range(len(pids)):
pid = pids[i]
cid = cids[i]
comment = comments[i]
text = comment[0]
created_at = comment[1]
author = comment[2]
# 对评论数据进行处理,如保存到数据库等操作
...
def closed(self, spider):
self.browser.close()
```
关于数据可视化分析,我们可以使用Python的数据科学库——Pandas和可视化库——Matplotlib,以下是一个简单的示例代码:
```
import pandas as pd
import matplotlib.pyplot as plt
# 读取数据
df = pd.read_csv('comment.csv', encoding='utf-8')
# 计算评论数量
comment_count = df.groupby('author').size().reset_index(name='comment_count')
# 绘制柱状图
plt.bar(comment_count['author'], comment_count['comment_count'])
plt.xticks(rotation=90)
plt.show()
# 统计评论时间分布
df['created_at'] = pd.to_datetime(df['created_at'], format='%a %b %d %H:%M:%S +0800 %Y')
df['hour'] = df['created_at'].apply(lambda x: x.hour)
comment_hour_count = df.groupby('hour').size().reset_index(name='comment_count')
# 绘制折线图
plt.plot(comment_hour_count['hour'], comment_hour_count['comment_count'])
plt.xticks(range(24))
plt.show()
```
此处我们假设已经将爬取到的数据保存到了一个comment.csv文件中。可视化分析部分,我们使用Pandas的groupby方法对数据进行统计,然后使用Matplotlib进行可视化绘制。如上代码片段中的示例,我们分别绘制了柱状图和折线图,用以展现评论数量的分布情况。具体的图表效果还需要根据实际数据和需求进行调整和优化。
相关推荐
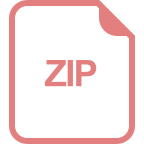
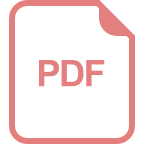
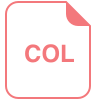
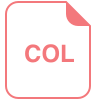










