运用简单C语言编写代码,设计一个算法统计在输入字符串总各个不同的频度并将结果存入文件(字符串中的合法字符为A~Z这36个字母和0~9这10个数字)
时间: 2024-10-24 08:13:37 浏览: 15
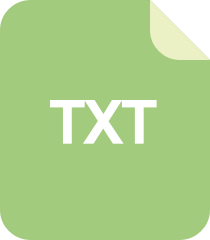
字符串中不同字符出现的频度

当然可以,我们可以使用C语言编写一个简单的程序来统计给定字符串中每个字符及其频率,并将结果保存到文件中。以下是一个基本的示例:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define ALPHABET_SIZE 36
#define NUMERIC_SIZE 10
typedef struct {
char ch;
int freq;
} CharFrequency;
void count_chars(char *str, CharFrequency* frequencies) {
for (int i = 0; str[i] != '\0'; i++) {
char ch = tolower(str[i]); // 转换为小写以合并大写和小写字母
if (isalpha(ch)) { // 判断是否为字母
ch -= 'a'; // 将字母转换为0-25的索引
frequencies[ch].freq++;
} else if (isdigit(ch)) { // 判断是否为数字
ch -= '0';
frequencies[ch].freq++;
}
}
}
void write_to_file(CharFrequency* frequencies, const char* filename) {
FILE* file = fopen(filename, "w");
if (file == NULL) {
printf("Failed to open file.\n");
return;
}
for (int i = 0; i <= ALPHABET_SIZE + NUMERIC_SIZE - 1; i++) {
if (frequencies[i].freq > 0) {
fprintf(file, "%c appears %d times.\n", 'a' + i, frequencies[i].freq);
}
}
fclose(file);
printf("Characters and their counts have been written to %s.\n", filename);
}
int main() {
char input[1000];
printf("Enter the string: ");
fgets(input, sizeof(input), stdin);
CharFrequency alpha_freq[ALPHABET_SIZE], num_freq[NUMERIC_SIZE];
memset(alpha_freq, 0, sizeof(alpha_freq));
memset(num_freq, 0, sizeof(num_freq));
count_chars(input, alpha_freq + 1); // 字符串中的第一个字母起始位置是1
count_chars(input, num_freq + 1); // 数字也是类似处理
char output_filename[50];
strcpy(output_filename, "char_counts.txt"); // 输出文件名
write_to_file(alpha_freq, output_filename);
write_to_file(num_freq, "num_counts.txt");
return 0;
}
```
当你运行这个程序并输入字符串后,它会计算出每个字母和数字的频率,并分别存储在一个文本文件(如 `char_counts.txt` 和 `num_counts.txt`)中。
阅读全文
相关推荐
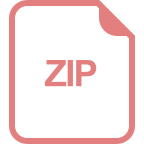
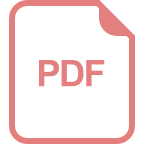






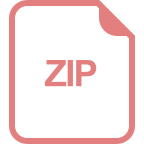
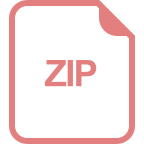
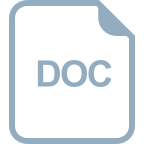
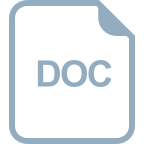
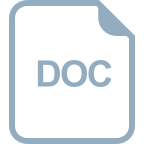




