将多个不同长、宽、高、重量的集装箱装入两个不同长、宽、高、载重限制的货船,并画出装载图。PYTHON
时间: 2023-09-30 17:05:40 浏览: 133
这是一个 NP-完全问题,也就是说没有多项式时间的解法。我们可以使用贪心算法来近似解决这个问题。以下是 Python 代码:
```python
class Container:
def __init__(self, length, width, height, weight):
self.length = length
self.width = width
self.height = height
self.weight = weight
class Ship:
def __init__(self, length, width, height, weight_limit):
self.length = length
self.width = width
self.height = height
self.weight_limit = weight_limit
self.containers = []
def load_container(self, container):
if (len(self.containers) == 0):
self.containers.append([container])
return True
for stack in self.containers:
if (self.can_load(stack, container)):
stack.append(container)
return True
if (self.can_load_new_stack(container)):
self.containers.append([container])
return True
return False
def can_load(self, stack, container):
if (sum(c.weight for c in stack) + container.weight > self.weight_limit):
return False
length = max(c.length for c in stack)
width = max(c.width for c in stack)
height = sum(c.height for c in stack) + container.height
if (length > self.length or width > self.width or height > self.height):
return False
return True
def can_load_new_stack(self, container):
if (container.weight > self.weight_limit or
container.length > self.length or
container.width > self.width or
container.height > self.height):
return False
return True
def load_containers(containers, ships):
ships.sort(key=lambda s: s.weight_limit, reverse=True)
for container in containers:
loaded = False
for ship in ships:
if (ship.load_container(container)):
loaded = True
break
if (not loaded):
return False
return True
# Example usage
c1 = Container(10, 5, 3, 2)
c2 = Container(8, 4, 4, 3)
c3 = Container(6, 3, 2, 1)
c4 = Container(7, 4, 5, 2)
c5 = Container(9, 6, 4, 3)
s1 = Ship(20, 8, 6, 10)
s2 = Ship(15, 6, 5, 7)
containers = [c1, c2, c3, c4, c5]
ships = [s1, s2]
if (load_containers(containers, ships)):
for i, ship in enumerate(ships):
print("Ship", i+1)
for j, stack in enumerate(ship.containers):
print("Stack", j+1)
for container in stack:
print(container.length, container.width, container.height, container.weight)
else:
print("Not enough space to load all containers")
```
这个算法的基本思路是,对于每个集装箱,我们尝试将其装入能够承载它的载重限制最大的船只中。如果没有可以承载它的船只,就返回 False。我们使用一个二维列表来存储每个船只中的集装箱,其中每个子列表表示一个货物堆。最后,我们输出每个船只中的所有货物堆和其中的集装箱。
阅读全文
相关推荐
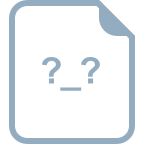
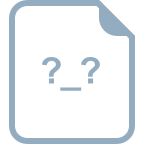
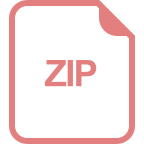
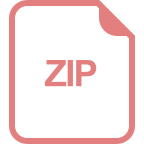
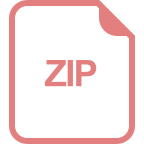
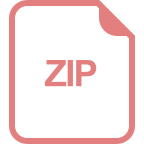
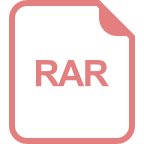
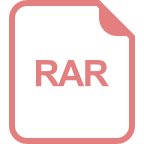
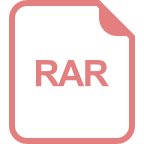
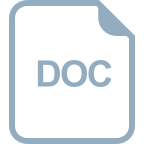
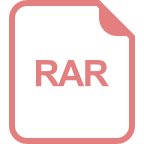
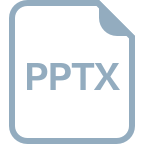
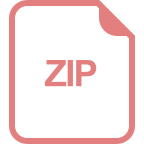
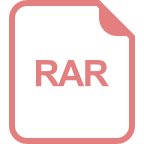
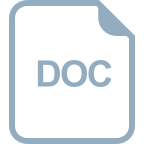
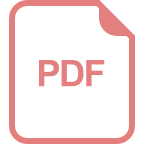
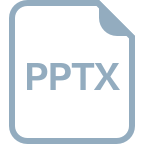