有一个正方形,已知正方形的右上角和左下角的坐标,有一条由若干个点组成的曲线 穿过这个正方形,求:如何用Java程序判断这个曲线在正方形中是左进右出还是右进上出还是下进上不出还是左进上不出右不出
时间: 2024-06-11 10:04:36 浏览: 165
可以采用射线法判断曲线在正方形中的走向。
具体步骤如下:
1. 获取正方形的四个顶点坐标,分别为A、B、C、D。
2. 将曲线的所有点按照横坐标排序,找到最左边和最右边的点,分别为P1和P2。
3. 将P1和P2连成一条直线,作为射线的方向。
4. 将射线从正方形的左侧边界向右侧延伸,统计射线与曲线的交点个数,记为count1。
5. 将射线从正方形的右侧边界向左侧延伸,统计射线与曲线的交点个数,记为count2。
6. 如果count1和count2均为奇数,则曲线是从左侧进入正方形,从右侧出去;如果count1和count2均为偶数,则曲线是从右侧进入正方形,从左侧出去。
7. 将射线从正方形的上侧边界向下延伸,统计射线与曲线的交点个数,记为count3。
8. 将射线从正方形的下侧边界向上延伸,统计射线与曲线的交点个数,记为count4。
9. 如果count3和count4均为奇数,则曲线是从下侧进入正方形,从上侧出去;如果count3和count4均为偶数,则曲线是从上侧进入正方形,从下侧出去。
10. 根据以上统计结果,可以判断曲线在正方形中的走向。
Java代码实现如下:
```
public static int countIntersection(Point p1, Point p2, List<Point> curve) {
int count = 0;
for (int i = 0; i < curve.size() - 1; i++) {
Point p3 = curve.get(i);
Point p4 = curve.get(i + 1);
if (isIntersect(p1, p2, p3, p4)) {
count++;
}
}
return count;
}
public static boolean isIntersect(Point p1, Point p2, Point p3, Point p4) {
double d1 = direction(p3, p4, p1);
double d2 = direction(p3, p4, p2);
double d3 = direction(p1, p2, p3);
double d4 = direction(p1, p2, p4);
if (((d1 > 0 && d2 < 0) || (d1 < 0 && d2 > 0)) && ((d3 > 0 && d4 < 0) || (d3 < 0 && d4 > 0))) {
return true;
} else if (d1 == 0 && onSegment(p3, p4, p1)) {
return true;
} else if (d2 == 0 && onSegment(p3, p4, p2)) {
return true;
} else if (d3 == 0 && onSegment(p1, p2, p3)) {
return true;
} else if (d4 == 0 && onSegment(p1, p2, p4)) {
return true;
}
return false;
}
public static double direction(Point p1, Point p2, Point p3) {
return (p3.x - p1.x) * (p2.y - p1.y) - (p2.x - p1.x) * (p3.y - p1.y);
}
public static boolean onSegment(Point p1, Point p2, Point p3) {
return Math.min(p1.x, p2.x) <= p3.x && p3.x <= Math.max(p1.x, p2.x) && Math.min(p1.y, p2.y) <= p3.y && p3.y <= Math.max(p1.y, p2.y);
}
public static String getCurveDirection(Point upperRight, Point lowerLeft, List<Point> curve) {
Point A = new Point(lowerLeft.x, upperRight.y);
Point B = new Point(upperRight.x, upperRight.y);
Point C = new Point(upperRight.x, lowerLeft.y);
Point D = new Point(lowerLeft.x, lowerLeft.y);
Point P1 = curve.get(0);
Point P2 = curve.get(curve.size() - 1);
int count1 = countIntersection(A, B, curve);
int count2 = countIntersection(B, A, curve);
int count3 = countIntersection(C, D, curve);
int count4 = countIntersection(D, C, curve);
if (count1 % 2 == 1 && count2 % 2 == 1) {
return "Left in, right out";
} else if (count1 % 2 == 0 && count2 % 2 == 0) {
return "Right in, left out";
} else if (count3 % 2 == 1 && count4 % 2 == 1) {
return "Down in, up out";
} else if (count3 % 2 == 0 && count4 % 2 == 0) {
return "Up in, down out";
} else {
return "Unknown";
}
}
```
其中,countIntersection方法用于统计射线与曲线的交点个数;isIntersect方法用于判断两条线段是否相交;direction方法用于计算向量的方向;onSegment方法用于判断点是否在线段上;getCurveDirection方法是主方法,用于判断曲线的走向。
阅读全文
相关推荐
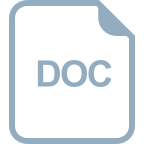
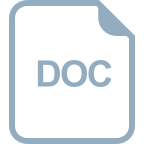
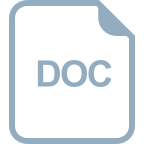


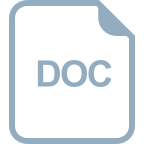
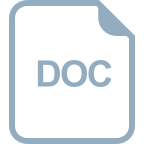
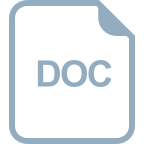
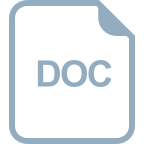
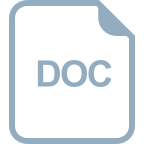
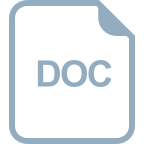
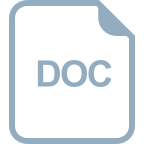
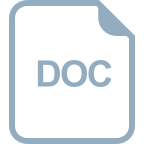
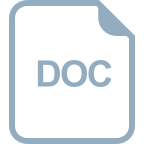
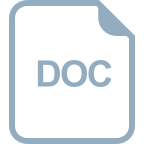
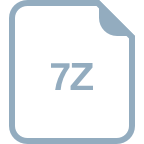
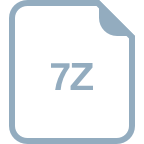
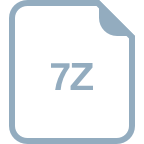
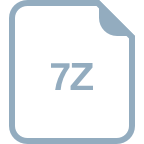
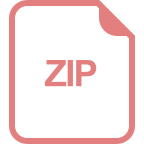