qt 不同线程发信号
时间: 2023-11-19 07:07:13 浏览: 32
在Qt中,不同线程之间发信号有两种常用的方法:
1. 使用Qt的信号槽机制:在不同线程的对象之间,可以通过连接信号和槽来实现通信。在发送信号时,Qt会自动将信号发送到接收槽所在的线程。要在不同线程之间发送信号,可以使用`Qt::QueuedConnection`连接模式,这将确保信号的执行发生在接收槽所在的线程上。
以下是一个示例代码,演示了如何在不同线程之间发送和接收信号:
```cpp
// 定义一个发送信号的类
class Sender : public QObject
{
Q_OBJECT
public:
void startSending()
{
QTimer* timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &Sender::sendSignal);
timer->start(1000);
}
signals:
void signalSent();
private:
void sendSignal()
{
emit signalSent();
}
};
// 定义一个接收信号的类
class Receiver : public QObject
{
Q_OBJECT
public slots:
void handleSignal()
{
qDebug() << "Signal received in thread: " << QThread::currentThreadId();
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 创建发送和接收对象
Sender sender;
Receiver receiver;
// 将发送者对象的信号连接到接收者对象的槽
QObject::connect(&sender, &Sender::signalSent, &receiver, &Receiver::handleSignal, Qt::QueuedConnection);
// 创建一个新线程,并将接收者对象移动到该线程
QThread thread;
receiver.moveToThread(&thread);
thread.start();
// 启动发送信号的逻辑
sender.startSending();
return app.exec();
}
```
在上述代码中,`Sender`类负责定时发送信号,`Receiver`类负责接收信号并处理。通过将`Receiver`对象移动到一个新线程中,我们可以模拟不同线程之间的通信。
2. 使用Qt的事件系统:Qt的事件系统允许在不同线程之间发送和接收自定义事件。要在不同线程之间发送事件,可以使用`QCoreApplication::postEvent()`函数。
以下是一个使用事件系统的示例代码:
```cpp
// 定义一个自定义事件
class CustomEvent : public QEvent
{
public:
CustomEvent() : QEvent(QEvent::Type(QEvent::User + 1)) {}
};
// 定义一个接收事件的类
class EventReceiver : public QObject
{
Q_OBJECT
protected:
bool event(QEvent* event) override
{
if (event->type() == QEvent::Type(QEvent::User + 1))
{
qDebug() << "Event received in thread: " << QThread::currentThreadId();
return true;
}
return QObject::event(event);
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 创建一个新线程,并将事件接收对象移动到该线程
QThread thread;
EventReceiver receiver;
receiver.moveToThread(&thread);
thread.start();
// 在不同线程中发送自定义事件
QCoreApplication::postEvent(&receiver, new CustomEvent());
return app.exec();
}
```
在上述代码中,`CustomEvent`类表示自定义事件,`EventReceiver`类继承自`QObject`,并重写了`event()`函数以处理自定义事件。通过将`EventReceiver`对象移动到一个新线程中,并使用`QCoreApplication::postEvent()`在不同线程之间发送自定义事件,我们可以实现跨线程的通信。
相关推荐
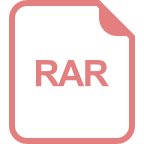
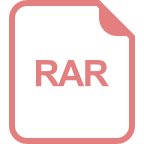
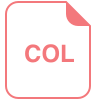
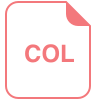
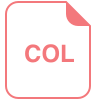
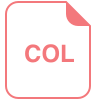
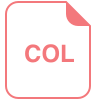









