实现一个通讯录和短信的管理系统
时间: 2024-03-25 09:39:11 浏览: 126
一个通讯录和短信管理系统需要实现以下基本功能:
1. 添加联系人
2. 查看联系人
3. 编辑联系人
4. 删除联系人
5. 发送短信
下面是一个使用Python实现简单通讯录和短信管理系统的示例代码:
```python
contacts = {}
def add_contact(name, phone_number, email):
contacts[name] = {"phone": phone_number, "email": email}
print("Contact added successfully.")
def view_contact(name):
if name in contacts:
print(f"Name: {name}")
print(f"Phone Number: {contacts[name]['phone']}")
print(f"Email: {contacts[name]['email']}")
else:
print("Contact not found.")
def edit_contact(name, phone_number, email):
if name in contacts:
contacts[name]["phone"] = phone_number
contacts[name]["email"] = email
print("Contact edited successfully.")
else:
print("Contact not found.")
def delete_contact(name):
if name in contacts:
del contacts[name]
print("Contact deleted successfully.")
else:
print("Contact not found.")
def send_sms(name, message):
if name in contacts:
phone_number = contacts[name]["phone"]
print(f"Sending message '{message}' to {name} ({phone_number})...")
# 在这里调用发送短信的API或模块
else:
print("Contact not found.")
while True:
print("1. Add contact")
print("2. View contact")
print("3. Edit contact")
print("4. Delete contact")
print("5. Send SMS")
print("6. Quit")
choice = input("Enter your choice: ")
if choice == "1":
name = input("Enter name: ")
phone_number = input("Enter phone number: ")
email = input("Enter email: ")
add_contact(name, phone_number, email)
elif choice == "2":
name = input("Enter name: ")
view_contact(name)
elif choice == "3":
name = input("Enter name: ")
phone_number = input("Enter phone number: ")
email = input("Enter email: ")
edit_contact(name, phone_number, email)
elif choice == "4":
name = input("Enter name: ")
delete_contact(name)
elif choice == "5":
name = input("Enter name: ")
message = input("Enter message: ")
send_sms(name, message)
elif choice == "6":
break
else:
print("Invalid choice. Please try again.")
```
这个示例代码在之前通讯录管理系统的基础上增加了发送短信的功能,实现方法是在send_sms函数中调用相应的API或模块来发送短信。用户可以通过输入联系人的姓名和短信内容来发送短信。
阅读全文
相关推荐
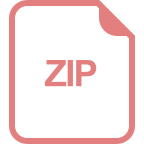
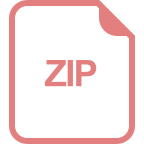


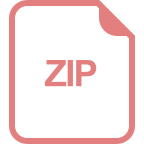
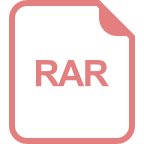
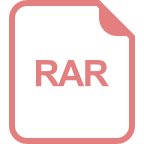
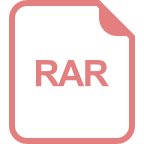
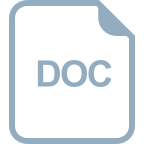
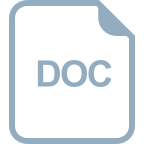
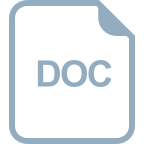
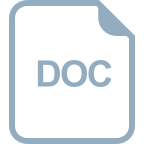
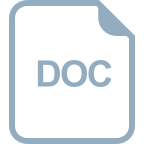
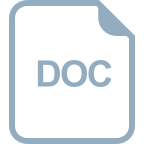
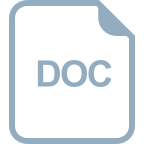
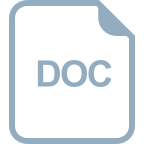