java两个用户分别向同一个账户存3000元 每次存1000 一共存三次
时间: 2024-02-18 17:01:54 浏览: 170
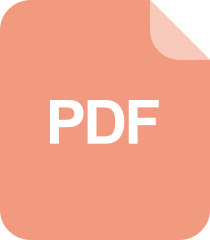
Java 多用户登录限制的实现方法

这是一个典型的多线程问题,可以使用Java的线程同步机制来解决。以下是一个简单的实现:
```java
class Account {
private int balance;
public Account(int balance) {
this.balance = balance;
}
public synchronized void deposit(int amount) {
for (int i = 0; i < 3; i++) {
try {
Thread.sleep(1000); // 模拟存款过程中的耗时操作
} catch (InterruptedException e) {
e.printStackTrace();
}
balance += amount;
System.out.println(Thread.currentThread().getName() + " 存款 " + amount + " 元,余额为 " + balance + " 元。");
}
}
}
class Depositor implements Runnable {
private Account account;
private int amount;
public Depositor(Account account, int amount) {
this.account = account;
this.amount = amount;
}
@Override
public void run() {
account.deposit(amount);
}
}
public class Main {
public static void main(String[] args) {
Account account = new Account(0);
Thread user1 = new Thread(new Depositor(account, 1000), "用户1");
Thread user2 = new Thread(new Depositor(account, 1000), "用户2");
user1.start();
user2.start();
}
}
```
上述代码中,`Account` 类表示账户,其中的 `deposit` 方法使用 `synchronized` 关键字实现了线程同步,保证了多个线程同时访问时的安全性。`Depositor` 类表示存款者,其 `run` 方法中调用 `Account` 的 `deposit` 方法进行存款。`Main` 类中创建了两个线程,分别表示两个用户,通过启动这两个线程来模拟多用户同时存款的场景。
阅读全文
相关推荐
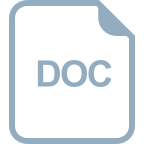
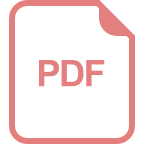


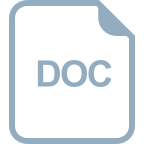








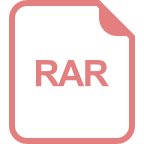
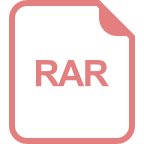
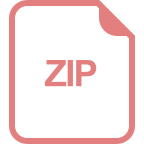

