用C语言写如下代码:基于Feistel结构构造一个分组密码算法。要求: 1.分组长度 = 128 bit 2.主密钥长度 >= 128 bit 3.轮数 >= 8 4.轮函数的设计要用到LFSR
时间: 2024-03-10 22:46:01 浏览: 37
以下是一个基于Feistel结构构造的简单分组密码算法的C语言代码,其分组长度为128位,主密钥长度为128位,轮数为8轮,轮函数采用了LFSR:
```c
#include <stdio.h>
#include <stdint.h>
#include <string.h>
#define ROUND_NUM 8
#define BLOCK_SIZE 16
typedef uint8_t u8;
typedef uint32_t u32;
typedef uint64_t u64;
u32 key[4] = {0x12345678, 0xabcdef01, 0x23456789, 0xabcdef01}; // 主密钥
u32 round_key[ROUND_NUM][2]; // 轮密钥
// 循环移位
u32 rotate_left(u32 x, int n) {
return (x << n) | (x >> (32 - n));
}
// LFSR
u32 lfsr(u32 x) {
return (x << 1) ^ ((x >> 31) & 0x00000001) ^ ((x >> 22) & 0x00000001) ^ ((x >> 2) & 0x00000001);
}
// 生成轮密钥
void generate_round_key() {
u32 lfsr_state = key[0] ^ key[1] ^ key[2] ^ key[3];
for (int i = 0; i < ROUND_NUM; i++) {
round_key[i][0] = lfsr_state;
round_key[i][1] = lfsr(lfsr_state);
lfsr_state = lfsr(lfsr_state);
}
}
// 轮函数
u32 round_function(u32 x, int round) {
u32 y = x ^ round_key[round][0];
y = (y + round_key[round][1]) % UINT32_MAX;
y = rotate_left(y, round);
y = lfsr(y);
return y;
}
// 加密函数
void encrypt(u8 *plaintext, u8 *ciphertext) {
u32 left = *(u32*)plaintext;
u32 right = *(u32*)(plaintext + 4);
for (int i = 0; i < ROUND_NUM; i++) {
u32 temp = left;
left = right;
right = temp ^ round_function(right, i);
}
*(u32*)ciphertext = right;
*(u32*)(ciphertext + 4) = left;
}
// 解密函数
void decrypt(u8 *ciphertext, u8 *plaintext) {
u32 left = *(u32*)ciphertext;
u32 right = *(u32*)(ciphertext + 4);
for (int i = ROUND_NUM - 1; i >= 0; i--) {
u32 temp = right;
right = left;
left = temp ^ round_function(left, i);
}
*(u32*)plaintext = left;
*(u32*)(plaintext + 4) = right;
}
int main() {
// 测试加密解密
u8 plaintext[BLOCK_SIZE] = {0x12, 0x34, 0x56, 0x78, 0xab, 0xcd, 0xef, 0x01,
0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 0x01};
u8 ciphertext[BLOCK_SIZE];
u8 decrypted_plaintext[BLOCK_SIZE];
generate_round_key();
encrypt(plaintext, ciphertext);
decrypt(ciphertext, decrypted_plaintext);
printf("Plaintext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x ", plaintext[i]);
}
printf("\nCiphertext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x ", ciphertext[i]);
}
printf("\nDecrypted plaintext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x ", decrypted_plaintext[i]);
}
printf("\n");
return 0;
}
```
在上述代码中,我们先定义了分组长度为128位及主密钥长度为128位,然后定义了轮数为8轮及轮函数采用的LFSR算法。接着在生成轮密钥时,我们将主密钥作为LFSR算法的初始状态,然后依次生成8个轮密钥。在加密时,我们采用了经典的Feistel结构,在每一轮中,将右半部分作为轮函数的输入,然后将输出与左半部分进行异或得到新的右半部分,最后将原来的左半部分作为新的右半部分。在解密时,我们只需要将加密过程中的轮函数逆序使用即可。
相关推荐
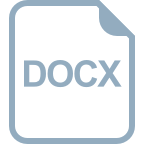
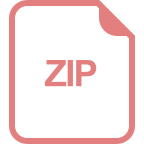
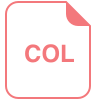
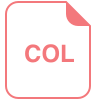
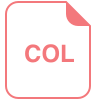
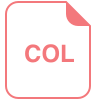
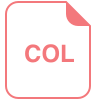









