js实现随机答题小游戏
时间: 2024-01-03 08:52:36 浏览: 81
以下是一个基于HTML、CSS和JavaScript的随机答题小游戏示例:
HTML代码:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>随机答题小游戏</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h1>随机答题小游戏</h1>
<div class="question">
<p id="question"></p>
<form id="answer-form">
<input type="text" id="answer-input" placeholder="请输入答案">
<button type="submit">提交</button>
</form>
<p id="result"></p>
</div>
<button id="next-btn">下一题</button>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS代码:
```
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
font-size: 32px;
font-weight: bold;
margin-bottom: 20px;
}
.question {
margin-bottom: 20px;
}
#question {
font-size: 24px;
margin-bottom: 10px;
}
#answer-form {
display: flex;
margin-bottom: 10px;
}
#answer-input {
flex: 1;
padding: 10px;
font-size: 16px;
border: none;
border-radius: 5px;
background-color: #f5f5f5;
}
#answer-input:focus {
outline: none;
}
button[type="submit"] {
padding: 10px;
font-size: 16px;
font-weight: bold;
color: #fff;
background-color: #007bff;
border: none;
border-radius: 5px;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: #0069d9;
}
#result {
font-size: 18px;
color: #dc3545;
margin-bottom: 10px;
}
#next-btn {
padding: 10px;
font-size: 16px;
font-weight: bold;
color: #fff;
background-color: #28a745;
border: none;
border-radius: 5px;
cursor: pointer;
}
#next-btn:hover {
background-color: #218838;
}
```
JavaScript代码:
```
// 定义题库数据
var questions = [
{
question: "1 + 1 = ?",
answer: "2"
},
{
question: "2 + 2 = ?",
answer: "4"
},
{
question: "3 + 3 = ?",
answer: "6"
},
{
question: "4 + 4 = ?",
answer: "8"
},
{
question: "5 + 5 = ?",
answer: "10"
},
{
question: "6 + 6 = ?",
answer: "12"
},
{
question: "7 + 7 = ?",
answer: "14"
},
{
question: "8 + 8 = ?",
answer: "16"
},
{
question: "9 + 9 = ?",
answer: "18"
},
{
question: "10 + 10 = ?",
answer: "20"
}
];
// 获取页面元素
var questionEl = document.getElementById("question");
var answerFormEl = document.getElementById("answer-form");
var answerInputEl = document.getElementById("answer-input");
var resultEl = document.getElementById("result");
var nextBtnEl = document.getElementById("next-btn");
// 定义当前题目索引
var currentQuestionIndex = null;
// 随机获取一个问题
function getRandomQuestion() {
var index = Math.floor(Math.random() * questions.length);
return questions[index];
}
// 显示问题
function showQuestion() {
var question = getRandomQuestion();
questionEl.textContent = question.question;
currentQuestionIndex = questions.indexOf(question);
}
// 处理答案提交事件
answerFormEl.addEventListener("submit", function(event) {
event.preventDefault();
var answer = answerInputEl.value.trim();
if (answer === questions[currentQuestionIndex].answer) {
resultEl.textContent = "回答正确!";
resultEl.style.color = "#28a745";
nextBtnEl.style.display = "block";
answerInputEl.disabled = true;
} else {
resultEl.textContent = "回答错误,请重新尝试。";
resultEl.style.color = "#dc3545";
}
});
// 处理下一题按钮点击事件
nextBtnEl.addEventListener("click", function() {
showQuestion();
resultEl.textContent = "";
resultEl.style.color = "#dc3545";
nextBtnEl.style.display = "none";
answerInputEl.value = "";
answerInputEl.disabled = false;
});
// 初始化游戏
showQuestion();
nextBtnEl.style.display = "none";
```
在这个示例中,我们首先定义了一个包含问题和答案的题库数据。然后,我们获取了页面元素,包括问题、答案输入框、结果提示和下一题按钮。接着,我们定义了一个随机获取问题的函数和一个显示问题的函数。在处理答案提交事件时,我们获取答案输入框的值,并与当前问题的答案进行比较。如果回答正确,我们将结果提示设置为“回答正确!”,并显示下一题按钮。如果回答错误,我们将结果提示设置为“回答错误,请重新尝试。”。在处理下一题按钮点击事件时,我们调用显示问题的函数,并重置结果提示和答案输入框。最后,我们在页面加载完成后调用显示问题的函数初始化游戏。
相关推荐
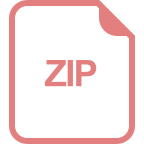
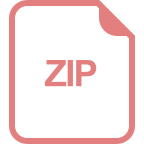














