pandas查询hive库
时间: 2023-11-09 17:59:08 浏览: 105
可以通过pyhive库来连接Hive并使用pandas进行查询,以下是示例代码:
```python
from pyhive import hive
import pandas as pd
# 连接Hive服务器
conn = hive.Connection(host='your_host', port=10000, username='your_username')
# 查询语句
query = 'SELECT * FROM your_database.your_table'
# 使用pandas读取查询结果
df = pd.read_sql(query, conn)
# 关闭连接
conn.close()
```
其中,'your_host'和'your_username'需要替换成你的Hive服务器地址和用户名,'your_database'和'your_table'需要替换成你要查询的数据库和表名。
相关问题
pandas将数据写入Hive的方法
可以使用PyHive库将pandas数据写入Hive。以下是一个示例代码:
```python
from pyhive import hive
import pandas as pd
# 创建连接
conn = hive.Connection(host='localhost', port=10000, username='hiveuser')
# 创建表
query = """
CREATE TABLE mytable (
col1 STRING,
col2 INT
)
"""
with conn.cursor() as cursor:
cursor.execute(query)
# 将pandas数据写入表
df = pd.DataFrame({'col1': ['foo', 'bar'], 'col2': [1, 2]})
with conn.cursor() as cursor:
cursor.execute("USE mydatabase")
cursor.execute("SET hive.exec.dynamic.partition.mode=nonstrict")
cursor.execute("SET hive.exec.max.dynamic.partitions=10000")
cursor.execute("SET hive.exec.max.dynamic.partitions.pernode=10000")
cursor.execute("SET hive.enforce.bucketing=true")
cursor.execute("SET hive.mapred.mode=nonstrict")
cursor.execute("SET hive.optimize.index.filter=true")
cursor.execute("SET hive.optimize.ppd=true")
cursor.execute("SET hive.vectorized.execution.enabled=true")
cursor.execute("SET hive.vectorized.execution.reduce.enabled=true")
cursor.execute("SET hive.vectorized.execution.reduce.groupby.enabled=true")
cursor.execute("SET hive.vectorized.execution.reduce.groupby.fixed.ordered=false")
cursor.execute("SET hive.vectorized.execution.reduce.groupby.variable.estimated=false")
cursor.execute("SET hive.vectorized.execution.reduce.groupby.variable.exact=false")
cursor.execute("SET hive.vectorized.execution.reduce.groupby.variable.force=false")
cursor.execute("SET hive.vectorized.execution.reduce.groupby.variable.width=32768")
cursor.execute("SET hive.vectorized.execution.row.filter.enabled=true")
cursor.execute("SET hive.vectorized.execution.row.filter.pushdown=true")
cursor.execute("SET hive.vectorized.groupby.checkinterval=4096")
cursor.execute("SET hive.cbo.enable=true")
cursor.execute("SET hive.stats.fetch.column.stats=true")
cursor.execute("SET hive.stats.fetch.partition.stats=true")
cursor.execute("SET hive.compute.query.using.stats=true")
cursor.execute("SET hive.stats.join.factor=1.0")
cursor.execute("SET hive.stats.key.prefix=stats_")
cursor.execute("SET hive.stats.ndv.error=0.05")
cursor.execute("SET hive.stats.reliable=true")
cursor.execute("SET hive.stats.autogather=true")
cursor.execute("SET hive.stats.autogather.interval=10000")
cursor.execute("SET hive.stats.autogather.maxsize=10000")
df.to_sql(name='mytable', con=conn, if_exists='append', index=False)
# 关闭连接
conn.close()
```
请注意,代码中的连接信息需要根据您的环境进行修改。另外,如果需要写入分区表,可以在`to_sql`方法中使用`partition_by`参数指定分区列。
spark将已经pandas读取出来的dataframe数据存入hive
在将 Pandas DataFrame 存储到 Hive 中之前,需要先将其转换为 Spark DataFrame。可以使用 PySpark 的 SQLContext 或 SparkSession 对象创建 Spark DataFrame。假设已经创建了一个名为 `pandas_df` 的 Pandas DataFrame,然后可以执行以下步骤将其存储到 Hive 中:
1. 导入必要的库和模块:
```python
from pyspark.sql import SparkSession, SQLContext
```
2. 创建 SparkSession 对象:
```python
spark = SparkSession.builder \
.appName("pandas_to_hive") \
.config("spark.sql.warehouse.dir", "/user/hive/warehouse") \
.enableHiveSupport() \
.getOrCreate()
```
其中,`appName` 是应用程序名称,`config` 指定了 Hive 数据仓库的路径,`enableHiveSupport` 用于启用 Hive 支持。
3. 将 Pandas DataFrame 转换为 Spark DataFrame:
```python
spark_df = spark.createDataFrame(pandas_df)
```
4. 将 Spark DataFrame 存储到 Hive 中:
```python
spark_df.write \
.mode("overwrite") \
.saveAsTable("database_name.table_name")
```
其中,`mode` 指定了写入模式,`saveAsTable` 将数据写入到指定的表中,如果表不存在,则会自动创建。
完整示例代码如下:
```python
from pyspark.sql import SparkSession, SQLContext
import pandas as pd
# 创建 SparkSession 对象
spark = SparkSession.builder \
.appName("pandas_to_hive") \
.config("spark.sql.warehouse.dir", "/user/hive/warehouse") \
.enableHiveSupport() \
.getOrCreate()
# 读取 Pandas DataFrame
pandas_df = pd.read_csv("path/to/csv/file.csv")
# 将 Pandas DataFrame 转换为 Spark DataFrame
spark_df = spark.createDataFrame(pandas_df)
# 将 Spark DataFrame 存储到 Hive 中
spark_df.write \
.mode("overwrite") \
.saveAsTable("database_name.table_name")
```
请根据实际情况修改代码中的参数和路径。
阅读全文
相关推荐
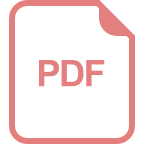
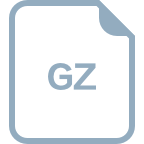













