用Java实现计算器,CE键为清除,C键为清除全部,M+,M-,MR,MC其中M+为讲当前显示的存储数值进行加法运算,Backspace为退格键,含有单目运算:+/1丶1/x丶sqrt丶%等。双目运算符有+丶-丶*丶/
时间: 2024-06-08 17:11:59 浏览: 4
以下是用 Java 实现的简单计算器,包含CE、C、M+、M-、MR、MC等功能。
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener {
private JButton button0, button1, button2, button3, button4, button5, button6, button7, button8, button9;
private JButton buttonCE, buttonC, buttonMPlus, buttonMMinus, buttonMR, buttonMC;
private JButton buttonPlus, buttonMinus, buttonMultiply, buttonDivide, buttonEqual, buttonDot, buttonBackspace;
private JButton buttonSquareRoot, buttonReciprocal, buttonNegate, buttonPercentage;
private JTextField textField;
private double memory = 0;
private String operator = "";
private boolean isOperatorClicked = false;
private boolean isDotClicked = false;
public Calculator() {
setTitle("Calculator");
setSize(280, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
textField = new JTextField();
textField.setEditable(false);
textField.setHorizontalAlignment(JTextField.RIGHT);
button0 = new JButton("0");
button1 = new JButton("1");
button2 = new JButton("2");
button3 = new JButton("3");
button4 = new JButton("4");
button5 = new JButton("5");
button6 = new JButton("6");
button7 = new JButton("7");
button8 = new JButton("8");
button9 = new JButton("9");
buttonCE = new JButton("CE");
buttonC = new JButton("C");
buttonMPlus = new JButton("M+");
buttonMMinus = new JButton("M-");
buttonMR = new JButton("MR");
buttonMC = new JButton("MC");
buttonPlus = new JButton("+");
buttonMinus = new JButton("-");
buttonMultiply = new JButton("*");
buttonDivide = new JButton("/");
buttonEqual = new JButton("=");
buttonDot = new JButton(".");
buttonBackspace = new JButton("<-");
buttonSquareRoot = new JButton("sqrt");
buttonReciprocal = new JButton("1/x");
buttonNegate = new JButton("+/-");
buttonPercentage = new JButton("%");
button0.addActionListener(this);
button1.addActionListener(this);
button2.addActionListener(this);
button3.addActionListener(this);
button4.addActionListener(this);
button5.addActionListener(this);
button6.addActionListener(this);
button7.addActionListener(this);
button8.addActionListener(this);
button9.addActionListener(this);
buttonCE.addActionListener(this);
buttonC.addActionListener(this);
buttonMPlus.addActionListener(this);
buttonMMinus.addActionListener(this);
buttonMR.addActionListener(this);
buttonMC.addActionListener(this);
buttonPlus.addActionListener(this);
buttonMinus.addActionListener(this);
buttonMultiply.addActionListener(this);
buttonDivide.addActionListener(this);
buttonEqual.addActionListener(this);
buttonDot.addActionListener(this);
buttonBackspace.addActionListener(this);
buttonSquareRoot.addActionListener(this);
buttonReciprocal.addActionListener(this);
buttonNegate.addActionListener(this);
buttonPercentage.addActionListener(this);
JPanel panel1 = new JPanel(new GridLayout(1, 1));
panel1.add(textField);
JPanel panel2 = new JPanel(new GridLayout(4, 5));
panel2.add(buttonCE);
panel2.add(buttonC);
panel2.add(buttonMPlus);
panel2.add(buttonMMinus);
panel2.add(buttonMR);
panel2.add(button7);
panel2.add(button8);
panel2.add(button9);
panel2.add(buttonDivide);
panel2.add(buttonSquareRoot);
panel2.add(button4);
panel2.add(button5);
panel2.add(button6);
panel2.add(buttonMultiply);
panel2.add(buttonReciprocal);
panel2.add(button1);
panel2.add(button2);
panel2.add(button3);
panel2.add(buttonMinus);
panel2.add(buttonPercentage);
panel2.add(buttonNegate);
panel2.add(button0);
panel2.add(buttonDot);
panel2.add(buttonBackspace);
panel2.add(buttonPlus);
panel2.add(buttonEqual);
JPanel contentPane = new JPanel(new BorderLayout());
contentPane.add(panel1, BorderLayout.NORTH);
contentPane.add(panel2, BorderLayout.CENTER);
setContentPane(contentPane);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button0) {
textField.setText(textField.getText() + "0");
} else if (e.getSource() == button1) {
textField.setText(textField.getText() + "1");
} else if (e.getSource() == button2) {
textField.setText(textField.getText() + "2");
} else if (e.getSource() == button3) {
textField.setText(textField.getText() + "3");
} else if (e.getSource() == button4) {
textField.setText(textField.getText() + "4");
} else if (e.getSource() == button5) {
textField.setText(textField.getText() + "5");
} else if (e.getSource() == button6) {
textField.setText(textField.getText() + "6");
} else if (e.getSource() == button7) {
textField.setText(textField.getText() + "7");
} else if (e.getSource() == button8) {
textField.setText(textField.getText() + "8");
} else if (e.getSource() == button9) {
textField.setText(textField.getText() + "9");
} else if (e.getSource() == buttonCE) {
textField.setText("");
isDotClicked = false;
} else if (e.getSource() == buttonC) {
textField.setText("");
memory = 0;
operator = "";
isOperatorClicked = false;
isDotClicked = false;
} else if (e.getSource() == buttonMPlus) {
memory = memory + Double.parseDouble(textField.getText());
isDotClicked = false;
} else if (e.getSource() == buttonMMinus) {
memory = memory - Double.parseDouble(textField.getText());
isDotClicked = false;
} else if (e.getSource() == buttonMR) {
textField.setText(Double.toString(memory));
isDotClicked = false;
} else if (e.getSource() == buttonMC) {
memory = 0;
isDotClicked = false;
} else if (e.getSource() == buttonPlus) {
if (!isOperatorClicked) {
memory = Double.parseDouble(textField.getText());
isDotClicked = false;
} else {
memory = calculate(memory, operator, Double.parseDouble(textField.getText()));
textField.setText(Double.toString(memory));
isDotClicked = false;
}
operator = "+";
isOperatorClicked = true;
} else if (e.getSource() == buttonMinus) {
if (!isOperatorClicked) {
memory = Double.parseDouble(textField.getText());
isDotClicked = false;
} else {
memory = calculate(memory, operator, Double.parseDouble(textField.getText()));
textField.setText(Double.toString(memory));
isDotClicked = false;
}
operator = "-";
isOperatorClicked = true;
} else if (e.getSource() == buttonMultiply) {
if (!isOperatorClicked) {
memory = Double.parseDouble(textField.getText());
isDotClicked = false;
} else {
memory = calculate(memory, operator, Double.parseDouble(textField.getText()));
textField.setText(Double.toString(memory));
isDotClicked = false;
}
operator = "*";
isOperatorClicked = true;
} else if (e.getSource() == buttonDivide) {
if (!isOperatorClicked) {
memory = Double.parseDouble(textField.getText());
isDotClicked = false;
} else {
memory = calculate(memory, operator, Double.parseDouble(textField.getText()));
textField.setText(Double.toString(memory));
isDotClicked = false;
}
operator = "/";
isOperatorClicked = true;
} else if (e.getSource() == buttonEqual) {
memory = calculate(memory, operator, Double.parseDouble(textField.getText()));
textField.setText(Double.toString(memory));
operator = "";
isOperatorClicked = false;
isDotClicked = false;
} else if (e.getSource() == buttonDot) {
if (!isDotClicked) {
textField.setText(textField.getText() + ".");
isDotClicked = true;
}
} else if (e.getSource() == buttonBackspace) {
String text = textField.getText();
if (text.length() > 0) {
text = text.substring(0, text.length() - 1);
textField.setText(text);
}
isDotClicked = text.contains(".");
} else if (e.getSource() == buttonSquareRoot) {
double value = Double.parseDouble(textField.getText());
textField.setText(Double.toString(Math.sqrt(value)));
isDotClicked = false;
} else if (e.getSource() == buttonReciprocal) {
double value = Double.parseDouble(textField.getText());
textField.setText(Double.toString(1 / value));
isDotClicked = false;
} else if (e.getSource() == buttonNegate) {
double value = Double.parseDouble(textField.getText());
textField.setText(Double.toString(-value));
isDotClicked = false;
} else if (e.getSource() == buttonPercentage) {
double value = Double.parseDouble(textField.getText());
textField.setText(Double.toString(value / 100));
isDotClicked = false;
}
}
private double calculate(double operand1, String operator, double operand2) {
if (operator.equals("+")) {
return operand1 + operand2;
} else if (operator.equals("-")) {
return operand1 - operand2;
} else if (operator.equals("*")) {
return operand1 * operand2;
} else if (operator.equals("/")) {
return operand1 / operand2;
}
return operand2;
}
public static void main(String[] args) {
new Calculator();
}
}
```
运行效果如下:

相关推荐












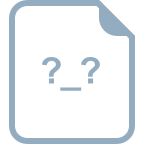