c++多线程 join
时间: 2023-12-08 09:39:36 浏览: 28
在C++中,join()函数用于等待一个线程完成其执行。如果一个线程被join()函数阻塞,那么它将一直等待,直到被阻塞的线程完成其执行为止。以下是一个使用join()函数的示例:
```c++
#include <iostream>
#include <thread>
using namespace std;
void test() {
printf("test start\n");
for (int i = 0; i < 10; i++) {
printf("test running\n");
}
printf("test end\n");
}
int main(void) {
cout << "The main start" << endl;
thread t1(test);
t1.join(); // 等待t1线程执行完毕
cout << "The main end" << endl;
return 0;
}
```
在上面的示例中,我们创建了一个名为t1的线程,并使用join()函数等待它完成执行。在t1线程执行完毕之前,主线程将一直被阻塞。当t1线程执行完毕后,主线程才会继续执行。
相关问题
C++多线程函数使用
C++多线程函数使用可以通过使用C++标准库中的<thread>头文件来实现。以下是使用多线程的基本步骤:
1. 包含头文件:<thread>
在使用多线程之前,需要包含<thread>头文件。
2. 创建线程对象
使用std::thread类创建一个线程对象,并将要执行的函数作为参数传递给它。例如:
```
void myFunction() {
// 线程要执行的代码
}
std::thread myThread(myFunction);
```
3. 启动线程
使用线程对象的成员函数start()来启动线程。例如:
```
myThread.start();
```
4. 等待线程结束
使用线程对象的成员函数join()来等待线程执行完毕。例如:
```
myThread.join();
```
这样就完成了一个简单的多线程函数的使用。需要注意的是,多线程编程需要注意线程间的同步和互斥,以避免竞态条件和数据访问冲突。
C++多线程编程
C++多线程编程是指利用C++语言的多线程库(如std::thread、pthread等)实现多线程程序。多线程编程可以提高程序的并行度,从而提高程序的性能。在C++中,可以使用多线程来处理计算密集型任务、I/O密集型任务、网络通信等任务。
下面是一个简单的C++多线程编程示例:
```
#include <iostream>
#include <thread>
void print_message() {
std::cout << "Hello, world!" << std::endl;
}
int main() {
std::thread t(print_message);
t.join();
return 0;
}
```
在这个示例中,我们使用std::thread创建了一个新的线程,并在新线程中执行了print_message函数。通过调用t.join(),我们等待新线程执行完毕,并回收线程资源。
相关推荐
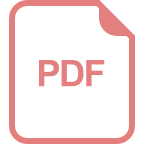
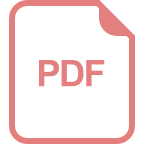












