已有两个数据集,如何进行数据清洗,数据挖掘,具体详细步骤及代码
时间: 2024-10-19 21:10:16 浏览: 29
数据清洗和数据挖掘是数据分析的重要步骤。以下是基本的步骤以及一些Python中的常用库示例:
**数据清洗步骤**:
1. **检查缺失值(Missing Values)**:使用pandas库的`isnull()`和`dropna()`函数查找和处理缺失值。可以选择删除含有缺失值的行(如果数据量不大),或者填充缺失值(如平均值、中位数、众数等)。
```python
import pandas as pd
df = pd.read_csv('data.csv')
df = df.dropna() # 删除所有含缺失值的行
```
2. **异常值检测(Outliers Detection)**:使用描述性统计和可视化工具,例如matplotlib或seaborn,找出离群点。
```python
import matplotlib.pyplot as plt
df.boxplot(column='column_name') # 查看箱线图找异常值
```
3. **标准化或归一化(Normalization or Scaling)**:对于数值型数据,可能需要将数据缩放到特定范围(0-1到1)或均值为0的标准差为1。
```python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
df['column_name'] = scaler.fit_transform(df['column_name'])
```
4. **一致性检查(Consistency Checks)**:检查数据类型是否一致,比如日期格式、类别编码等。
```python
df.dtypes # 查看每列的数据类型
```
**数据挖掘步骤**:
1. **探索性数据分析(Exploratory Data Analysis, EDA)**:使用matplotlib, seaborn等绘制图表,了解数据分布、相关性等。
```python
df.plot(kind='scatter', x='column1', y='column2')
```
2. **特征工程(Feature Engineering)**:根据业务需求,创建新的特征,如组合现有特征、转换非数值特征等。
3. **选择模型(Model Selection)**:根据问题类型(回归、分类、聚类等)选择合适的算法,如sklearn库中有许多预训练好的模型。
```python
from sklearn.linear_model import LinearRegression
model = LinearRegression()
```
4. **训练模型(Train the Model)**:将数据分为训练集和测试集,用训练集训练模型。
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
model.fit(X_train, y_train)
```
5. **评估模型(Model Evaluation)**:使用交叉验证或其他方法评估模型性能,如准确率、精确率、召回率等。
```python
from sklearn.metrics import accuracy_score
accuracy = accuracy_score(y_test, model.predict(X_test))
```
6. **优化模型(Model Tuning)**:调整模型参数以提高性能,例如网格搜索或随机搜索。
```python
from sklearn.model_selection import GridSearchCV
param_grid = {'parameter': [value1, value2]}
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X_train, y_train)
```
完成以上步骤后,记得定期保存中间结果和最终模型,以便于后续分析或复现实验。
阅读全文
相关推荐
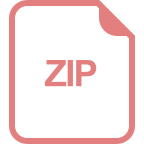
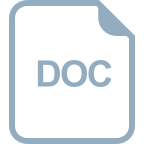
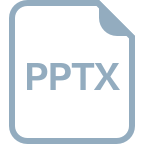















