用devc++制作贪吃蛇游戏
时间: 2023-08-17 08:04:23 浏览: 109
好的,我可以为您提供一些基础代码,您可以在Dev-C++中进行修改和调试。
```c++
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define MAX_X 50 // 地图的宽度
#define MAX_Y 20 // 地图的高度
// 定义一个结构体表示蛇的每个节点
struct Node {
int x; // 节点的横坐标
int y; // 节点的纵坐标
struct Node *next; // 指向下一个节点的指针
};
// 定义一个结构体表示食物
struct Food {
int x; // 食物的横坐标
int y; // 食物的纵坐标
};
// 定义一个枚举类型表示蛇的运动方向
enum Direct {
UP,
DOWN,
LEFT,
RIGHT
};
int length = 3; // 蛇的长度
struct Node *head; // 蛇头指针
struct Node *tail; // 蛇尾指针
struct Food food; // 食物
enum Direct direct; // 蛇的运动方向
int score = 0; // 分数
// 初始化地图
void init() {
// 隐藏光标
CONSOLE_CURSOR_INFO cursor_info = { 1, 0 };
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
// 随机生成食物的位置
srand((unsigned int)time(NULL));
food.x = rand() % MAX_X;
food.y = rand() % MAX_Y;
// 初始化蛇的位置
head = (struct Node *)malloc(sizeof(struct Node));
head->x = MAX_X / 2;
head->y = MAX_Y / 2;
head->next = NULL;
tail = head;
for (int i = 1; i < length; i++) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->x = head->x + i;
node->y = head->y;
node->next = NULL;
tail->next = node;
tail = node;
}
// 初始化蛇的运动方向
direct = RIGHT;
}
// 在屏幕上画出地图
void drawMap() {
system("cls"); // 清空屏幕
for (int i = 0; i <= MAX_X + 1; i++) {
printf("#");
}
printf("\n");
for (int i = 0; i < MAX_Y; i++) {
printf("#");
for (int j = 0; j < MAX_X; j++) {
if (i == head->y && j == head->x) { // 如果是蛇头
printf("@");
} else if (i == food.y && j == food.x) { // 如果是食物
printf("*");
} else {
bool flag = false;
struct Node *p = head->next;
while (p != NULL) { // 如果是蛇身
if (p->x == j && p->y == i) {
printf("#");
flag = true;
break;
}
p = p->next;
}
if (!flag) {
printf(" ");
}
}
}
printf("#");
printf("\n");
}
for (int i = 0; i <= MAX_X + 1; i++) {
printf("#");
}
printf("\n");
printf("Score: %d\n", score);
}
// 判断蛇是否吃到了食物
bool isEatFood() {
return head->x == food.x && head->y == food.y;
}
// 在屏幕上画出食物
void drawFood() {
food.x = rand() % MAX_X;
food.y = rand() % MAX_Y;
struct Node *p = head;
while (p != NULL) {
if (food.x == p->x && food.y == p->y) { // 如果食物与蛇身重合,重新生成
food.x = rand() % MAX_X;
food.y = rand() % MAX_Y;
p = head;
continue;
}
p = p->next;
}
gotoxy(food.x + 1, food.y + 1);
printf("*");
}
// 蛇的运动
void move() {
struct Node *newHead = (struct Node *)malloc(sizeof(struct Node));
newHead->x = head->x;
newHead->y = head->y;
switch (direct) {
case UP:
newHead->y--;
break;
case DOWN:
newHead->y++;
break;
case LEFT:
newHead->x--;
break;
case RIGHT:
newHead->x++;
break;
}
newHead->next = head;
head = newHead;
if (!isEatFood()) {
struct Node *p = head;
while (p->next->next != NULL) {
p = p->next;
}
tail = p;
free(p->next);
p->next = NULL;
} else {
length++;
score++;
drawFood();
}
}
// 判断游戏是否结束
bool isGameOver() {
if (head->x < 0 || head->x >= MAX_X || head->y < 0 || head->y >= MAX_Y) { // 是否撞墙
return true;
}
struct Node *p = head->next;
while (p != NULL) { // 是否撞到自己的身体
if (head->x == p->x && head->y == p->y) {
return true;
}
p = p->next;
}
return false;
}
// 控制蛇的运动方向
void control() {
if (_kbhit()) {
char ch = _getch();
if (ch == 'w' || ch == 'W') {
if (direct != DOWN) {
direct = UP;
}
} else if (ch == 's' || ch == 'S') {
if (direct != UP) {
direct = DOWN;
}
} else if (ch == 'a' || ch == 'A') {
if (direct != RIGHT) {
direct = LEFT;
}
} else if (ch == 'd' || ch == 'D') {
if (direct != LEFT) {
direct = RIGHT;
}
}
}
}
int main() {
init(); // 初始化地图
drawMap(); // 画出地图
while (!isGameOver()) {
control(); // 控制蛇的运动方向
move(); // 蛇的运动
drawMap(); // 画出地图
Sleep(100); // 控制蛇的速度
}
printf("Game Over!");
return 0;
}
```
这是一个基础版本的贪吃蛇游戏,您可以根据自己的需求进行修改和扩展。祝您编写愉快!
阅读全文
相关推荐
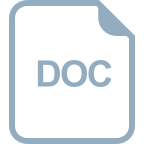
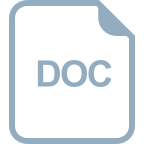
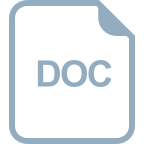
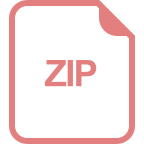
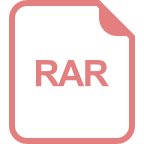
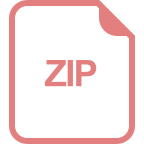
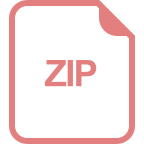
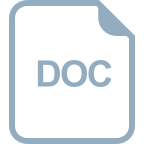
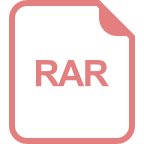
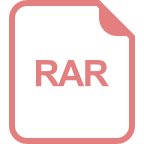
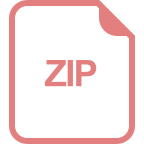
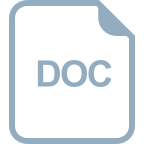
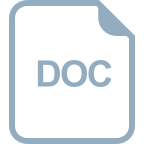
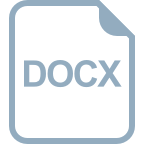
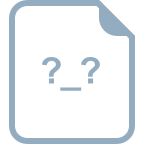