同时开启两个线程。共同获取1-100之间的所有数字,要求:将输出所有的奇数
时间: 2024-04-30 16:18:45 浏览: 17
可以使用Python内置的 `threading` 模块实现多线程,具体实现如下:
```python
import threading
# 定义一个函数,用于输出1-100之间的奇数
def print_odd_numbers():
for num in range(1, 101, 2):
print(num)
# 创建两个线程,分别执行输出奇数的函数
thread1 = threading.Thread(target=print_odd_numbers)
thread2 = threading.Thread(target=print_odd_numbers)
# 启动线程
thread1.start()
thread2.start()
# 等待线程执行完毕
thread1.join()
thread2.join()
```
以上代码中,我们定义了一个函数 `print_odd_numbers`,用于输出1-100之间的奇数。然后创建了两个线程,分别执行该函数。最后启动线程,等待线程执行完毕。在执行过程中,由于两个线程是并发执行的,因此输出的结果可能会有交错,但是所有的奇数都会被输出。
相关问题
同时开启两个线程。共同获取1-100之间的所有数字,要求:将输出所有的奇数用Java
可以使用 Java 的多线程编程来实现该需求。下面是一个简单的示例代码:
```java
public class OddNumberPrinter implements Runnable {
private int start;
private int end;
public OddNumberPrinter(int start, int end) {
this.start = start;
this.end = end;
}
public void run() {
for (int i = start; i <= end; i++) {
if (i % 2 == 1) {
System.out.println(i);
}
}
}
public static void main(String[] args) {
Thread t1 = new Thread(new OddNumberPrinter(1, 50));
Thread t2 = new Thread(new OddNumberPrinter(51, 100));
t1.start();
t2.start();
}
}
```
在该示例代码中,我们定义了一个 `OddNumberPrinter` 类实现 `Runnable` 接口,并在 `run()` 方法中循环遍历从 `start` 到 `end` 的所有数字,判断是否为奇数并输出。然后在 `main()` 方法中创建了两个线程,分别传入不同的范围参数,并调用 `start()` 方法启动线程。最终会输出从 1 到 100 之间的所有奇数。
创建两个线程 一个输出1-100的奇数,一个输出1-100的偶数 交替输出
可以使用线程和锁来实现交替输出奇偶数的功能。下面是一个示例代码:
```python
import threading
# 创建一个锁
lock = threading.Lock()
# 奇数线程
def odd_thread():
for i in range(1, 101, 2):
lock.acquire() # 获取锁
print(i)
lock.release() # 释放锁
# 偶数线程
def even_thread():
for i in range(2, 101, 2):
lock.acquire() # 获取锁
print(i)
lock.release() # 释放锁
# 创建并启动线程
t1 = threading.Thread(target=odd_thread)
t2 = threading.Thread(target=even_thread)
t1.start()
t2.start()
t1.join()
t2.join()
```
这个代码中,我们首先创建了一个锁对象 `lock`,然后定义了奇数线程函数 `odd_thread` 和偶数线程函数 `even_thread`。在每个线程的循环中,首先通过 `lock.acquire()` 获取锁,然后输出对应的数字,最后通过 `lock.release()` 释放锁。
最后,我们创建并启动两个线程 `t1` 和 `t2`,并使用 `t1.join()` 和 `t2.join()` 来等待两个线程的结束。这样,两个线程就会交替输出奇偶数。
相关推荐
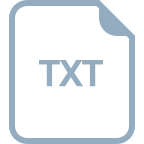
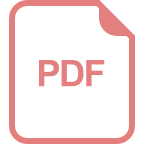












