从键盘输入3个正整数ab.c,其中c为 一个4位数,将ab的值交换后输出:将 c的值逆序输出,如:假设c的值为1234 逆序输出后,为4321 用C语言
时间: 2024-09-26 09:13:13 浏览: 18
在C语言中,你可以通过以下几个步骤实现这个功能:
1. 首先,我们需要从用户那里获取三个正整数a、b以及一个四位数c,并存储它们。可以使用`scanf`函数来实现输入。
```c
int a, b, c;
scanf("%d.%d.%d", &a, &b, &c);
```
2. 接着,我们将ab的值交换,这里可以用临时变量存储其中一个数。
```c
int temp = a;
a = b;
b = temp;
printf("交换后的ab: a=%d, b=%d\n", a, b);
```
3. 对c的四位数字进行逆序处理。我们可以将其转换成字符串,然后逐字符读取并反转顺序,最后再转换回整数。
```c
char str[5] = {0};
sprintf(str, "%d", c);
for (int i = 0, j = strlen(str) - 1; i < j; ++i, --j) {
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
int reversed_c = atoi(str);
printf("逆序后的c: %d\n", reversed_c);
```
完整的代码示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
int a, b, c;
scanf("%d.%d.%d", &a, &b, &c);
int temp = a;
a = b;
b = temp;
printf("交换后的ab: a=%d, b=%d\n", a, b);
char str[5] = {0};
sprintf(str, "%d", c);
for (int i = 0, j = strlen(str) - 1; i < j; ++i, --j) {
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
int reversed_c = atoi(str);
printf("逆序后的c: %d\n", reversed_c);
return 0;
}
```
相关推荐
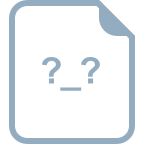
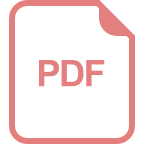
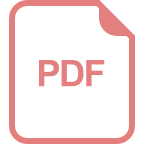



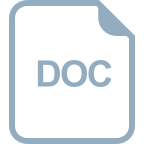
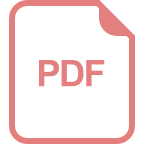
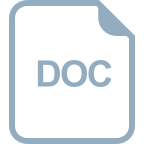
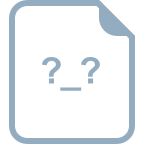
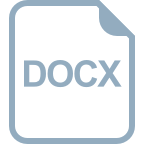
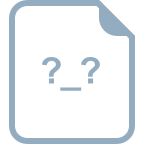
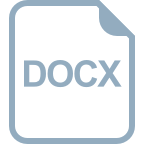
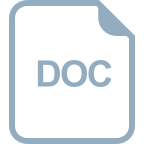
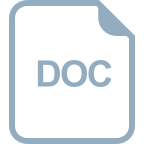