基于MFC的小游戏中鼠标拖拽图片的头文件和cpp文件
时间: 2024-02-27 09:56:08 浏览: 84
以下是一个基于 MFC 的小游戏中鼠标拖拽图片的头文件和 cpp 文件的示例:
头文件 DragImage.h:
```cpp
#pragma once
class CDragImage : public CStatic
{
public:
CDragImage();
virtual ~CDragImage();
BOOL LoadImage(UINT uResID);
void SetDropTarget(CWnd* pTarget);
void SetDragCursor(HCURSOR hCursor);
void SetDragImageOffset(CPoint ptOffset);
protected:
afx_msg void OnLButtonDown(UINT nFlags, CPoint point);
afx_msg void OnMouseMove(UINT nFlags, CPoint point);
afx_msg void OnLButtonUp(UINT nFlags, CPoint point);
DECLARE_MESSAGE_MAP()
private:
CBitmap m_bitmap;
CWnd* m_pDropTarget;
HCURSOR m_hCursor;
CPoint m_ptOffset;
BOOL m_bDragging;
};
```
cpp 文件 DragImage.cpp:
```cpp
#include "stdafx.h"
#include "DragImage.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
IMPLEMENT_DYNAMIC(CDragImage, CStatic)
BEGIN_MESSAGE_MAP(CDragImage, CStatic)
ON_WM_LBUTTONDOWN()
ON_WM_MOUSEMOVE()
ON_WM_LBUTTONUP()
END_MESSAGE_MAP()
CDragImage::CDragImage()
: m_pDropTarget(NULL)
, m_hCursor(NULL)
, m_bDragging(FALSE)
{
}
CDragImage::~CDragImage()
{
}
BOOL CDragImage::LoadImage(UINT uResID)
{
HBITMAP hBitmap = (HBITMAP)::LoadImage(AfxGetInstanceHandle(), MAKEINTRESOURCE(uResID), IMAGE_BITMAP, 0, 0, LR_DEFAULTCOLOR);
if (hBitmap == NULL)
{
return FALSE;
}
m_bitmap.Attach(hBitmap);
SetBitmap(hBitmap);
return TRUE;
}
void CDragImage::SetDropTarget(CWnd* pTarget)
{
m_pDropTarget = pTarget;
}
void CDragImage::SetDragCursor(HCURSOR hCursor)
{
m_hCursor = hCursor;
}
void CDragImage::SetDragImageOffset(CPoint ptOffset)
{
m_ptOffset = ptOffset;
}
void CDragImage::OnLButtonDown(UINT nFlags, CPoint point)
{
if (m_bitmap.GetSafeHandle() == NULL)
{
return;
}
m_bDragging = TRUE;
CRect rect;
GetWindowRect(&rect);
CDC* pDC = GetDC();
CDC memDC;
memDC.CreateCompatibleDC(pDC);
CBitmap* pOldBitmap = memDC.SelectObject(&m_bitmap);
CPoint ptImage(point - rect.TopLeft() - m_ptOffset);
if (m_hCursor != NULL)
{
::SetCursor(m_hCursor);
}
if (m_pDropTarget != NULL)
{
m_pDropTarget->SetCapture();
}
if (::ImageList_BeginDrag(NULL, 0, 0, 0))
{
::ImageList_AddMasked(NULL, m_bitmap, RGB(255, 0, 255));
::ImageList_DragEnter(NULL, point.x, point.y);
::ImageList_DragMove(point.x, point.y);
}
memDC.SelectObject(pOldBitmap);
ReleaseDC(pDC);
}
void CDragImage::OnMouseMove(UINT nFlags, CPoint point)
{
if (m_bDragging)
{
if (m_hCursor != NULL)
{
::SetCursor(m_hCursor);
}
if (::ImageList_DragMove(point.x, point.y))
{
if (m_pDropTarget != NULL)
{
CRect rect;
m_pDropTarget->GetWindowRect(&rect);
if (rect.PtInRect(point))
{
::ImageList_DragEnter(m_pDropTarget->GetSafeHwnd(), point.x, point.y);
}
else
{
::ImageList_DragLeave(m_pDropTarget->GetSafeHwnd());
}
}
}
}
}
void CDragImage::OnLButtonUp(UINT nFlags, CPoint point)
{
if (m_bDragging)
{
if (::ImageList_DragLeave(NULL))
{
if (m_pDropTarget != NULL)
{
CRect rect;
m_pDropTarget->GetWindowRect(&rect);
if (rect.PtInRect(point))
{
::ImageList_DragEnter(m_pDropTarget->GetSafeHwnd(), point.x, point.y);
::ImageList_Drop(m_pDropTarget->GetSafeHwnd(), point);
}
}
}
if (m_pDropTarget != NULL)
{
m_pDropTarget->ReleaseCapture();
}
::ImageList_EndDrag();
m_bDragging = FALSE;
}
}
```
注意:该示例代码仅供参考,请根据自己的实际需求进行修改和调整。
阅读全文
相关推荐
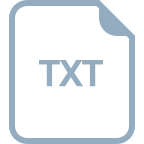
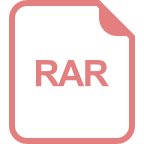
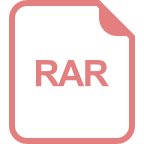
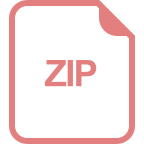
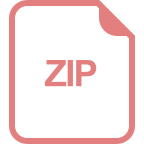
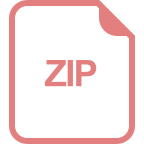
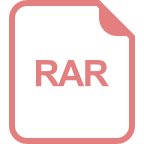
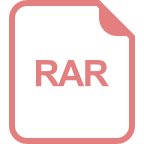
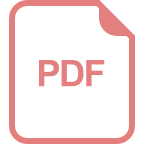
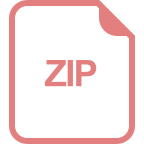
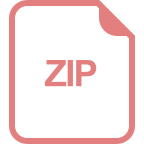