购物车javaweb
时间: 2024-12-05 07:14:12 浏览: 7
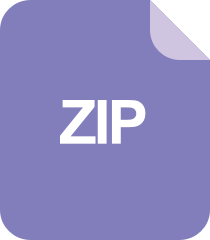
JavaWeb 购物车系统

在Java Web开发中,购物车是一个常见的功能模块,用于存储用户在购物网站或应用程序中选择购买的商品。购物车通常包括以下功能:
1. **添加商品到购物车**:用户可以浏览商品列表并选择将商品添加到购物车。
2. **查看购物车**:用户可以查看购物车中已添加的商品列表,包括商品名称、数量、价格等信息。
3. **修改商品数量**:用户可以修改购物车中每个商品的数量。
4. **删除商品**:用户可以从购物车中删除不需要的商品。
5. **结算**:用户可以查看购物车的总价并进行结算。
实现购物车的关键在于如何存储和管理用户的选择。常见的实现方式有以下几种:
### 1. 使用Session存储购物车
Session是服务器端存储用户会话信息的一种机制。每次用户添加商品到购物车时,可以将商品信息存储在Session中。这种方式适用于用户未登录的情况。
```java
// 添加商品到购物车
HttpSession session = request.getSession();
Map<String, Integer> cart = (Map<String, Integer>) session.getAttribute("cart");
if (cart == null) {
cart = new HashMap<>();
}
String productId = request.getParameter("productId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
cart.put(productId, quantity);
session.setAttribute("cart", cart);
```
### 2. 使用数据库存储购物车
如果用户已登录,可以将购物车信息存储在数据库中。这样用户在不同设备上登录时可以看到相同的购物车内容。
```sql
-- 创建购物车表
CREATE TABLE cart (
id INT PRIMARY KEY AUTO_INCREMENT,
user_id INT,
product_id INT,
quantity INT,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (product_id) REFERENCES products(id)
);
```
```java
// 添加商品到购物车
int userId = ...; // 获取当前用户ID
int productId = Integer.parseInt(request.getParameter("productId"));
int quantity = Integer.parseInt(request.getParameter("quantity"));
// 检查购物车中是否已有该商品
String sql = "SELECT * FROM cart WHERE user_id = ? AND product_id = ?";
PreparedStatement pstmt = connection.prepareStatement(sql);
pstmt.setInt(1, userId);
pstmt.setInt(2, productId);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
// 更新数量
int newQuantity = rs.getInt("quantity") + quantity;
String updateSql = "UPDATE cart SET quantity = ? WHERE id = ?";
PreparedStatement updatePstmt = connection.prepareStatement(updateSql);
updatePstmt.setInt(1, newQuantity);
updatePstmt.setInt(2, rs.getInt("id"));
updatePstmt.executeUpdate();
} else {
// 插入新记录
String insertSql = "INSERT INTO cart (user_id, product_id, quantity) VALUES (?, ?, ?)";
PreparedStatement insertPstmt = connection.prepareStatement(insertSql);
insertPstmt.setInt(1, userId);
insertPstmt.setInt(2, productId);
insertPstmt.setInt(3, quantity);
insertPstmt.executeUpdate();
}
```
### 3. 使用Cookie存储购物车
Cookie是存储在客户端的小数据块,可以用来存储购物车信息。不过,由于Cookie的大小限制和安全性问题,这种方式不太常用。
```java
// 添加商品到购物车
String productId = request.getParameter("productId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
Cookie[] cookies = request.getCookies();
Cookie cartCookie = null;
for (Cookie cookie : cookies) {
if (cookie.getName().equals("cart")) {
cartCookie = cookie;
break;
}
}
if (cartCookie == null) {
cartCookie = new Cookie("cart", productId + ":" + quantity);
} else {
String cart = cartCookie.getValue();
String[] items = cart.split(",");
boolean found = false;
for (int i = 0; i < items.length; i++) {
String[] item = items[i].split(":");
if (item[0].equals(productId)) {
items[i] = productId + ":" + (Integer.parseInt(item[1]) + quantity);
found = true;
break;
}
}
if (!found) {
cartCookie.setValue(cart + "," + productId + ":" + quantity);
} else {
cartCookie.setValue(String.join(",", items));
}
}
cartCookie.setPath("/");
response.addCookie(cartCookie);
```
阅读全文
相关推荐
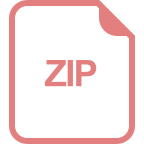
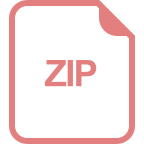
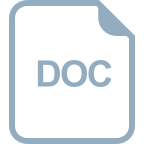

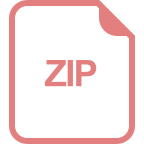
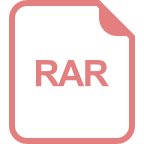
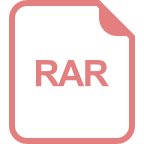
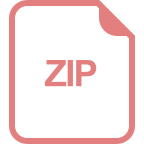





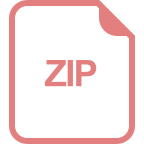
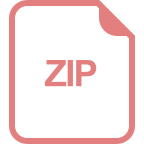