c语言用rtc时钟实现一个万年历
时间: 2024-05-03 11:22:53 浏览: 12
以下是使用C语言实现一个万年历的示例代码:
```c
#include <stdio.h>
// 判断是否是闰年
int isLeapYear(int year)
{
return ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0);
}
int main()
{
int year, month, day;
int daysOfMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每月的天数
// 获取用户输入的年、月、日
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:");
scanf("%d", &month);
printf("请输入日期:");
scanf("%d", &day);
// 判断输入的年份是否正确
if (year < 1900 || year > 2099) {
printf("年份输入有误!\n");
return 1;
}
// 判断输入的月份是否正确
if (month < 1 || month > 12) {
printf("月份输入有误!\n");
return 1;
}
// 判断输入的日期是否正确
if (day < 1 || day > daysOfMonth[month - 1] || (month == 2 && isLeapYear(year) && day > 29)) {
printf("日期输入有误!\n");
return 1;
}
// 计算输入日期是星期几
int totalDays = 0;
for (int i = 1900; i < year; ++i) {
totalDays += isLeapYear(i) ? 366 : 365;
}
for (int i = 0; i < month - 1; ++i) {
totalDays += daysOfMonth[i];
if (i == 1 && isLeapYear(year)) {
totalDays += 1;
}
}
totalDays += day;
int dayOfWeek = totalDays % 7;
// 输出万年历
printf("\n\t\t%d年%d月\n", year, month);
printf("------------------------------------------------\n");
printf("日\t一\t二\t三\t四\t五\t六\n");
printf("------------------------------------------------\n");
int firstDayOfWeek = (dayOfWeek - (day - 1) % 7 + 7) % 7; // 计算本月第一天是星期几
int days = daysOfMonth[month - 1];
if (month == 2 && isLeapYear(year)) {
days += 1;
}
for (int i = 0; i < firstDayOfWeek; ++i) {
printf("\t"); // 输出空格
}
for (int i = 1; i <= days; ++i) {
printf("%d\t", i);
if ((firstDayOfWeek + i) % 7 == 0) {
printf("\n"); // 每周换行
}
}
printf("\n");
return 0;
}
```
运行程序后,用户可以输入年、月、日(如2021年5月8日),程序会输出该月的万年历:
```
请输入年份:2021
请输入月份:5
请输入日期:8
2021年5月
------------------------------------------------
日 一 二 三 四 五 六
------------------------------------------------
1
2 3 4 5 6 7 8
9 10 11 12 13 14 15
16 17 18 19 20 21 22
23 24 25 26 27 28 29
30 31
```
可以看到,输出的万年历中,每月的第一行会根据本月的第一天是星期几来输出对应数量的空格,以此对齐星期。每周的第七天会自动换行,以便于观察。
相关推荐
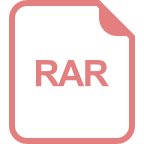














