Point类添加一个带参数的构造方法,传两个参数用于初始化坐标
时间: 2023-05-21 14:02:13 浏览: 122
好的,可以这样实现:
```java
public class Point {
private int x;
private int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
// 其它方法...
}
```
这样就可以通过传入两个参数来初始化 Point 对象的坐标了。
相关问题
定义一个名为Point的类模拟平面坐标的一个点。一个点可用x和y坐标描述。为该类定义一个无参数构造方法,用它创建坐标原点。定义一个带两个参数的构造方法,用它创建任意点。为两个成员定义setter方法和getter方法。完整程序
当然,这里是一个简单的`Point`类的示例,它满足你的需求:
```java
public class Point {
private double x;
private double y;
// 无参数构造方法,用于创建坐标原点
public Point() {
this.x = 0.0;
this.y = 0.0;
}
// 带两个参数的构造方法,用于创建任意点
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// getter方法获取x坐标
public double getX() {
return x;
}
// setter方法设置x坐标
public void setX(double x) {
this.x = x;
}
// getter方法获取y坐标
public double getY() {
return y;
}
// setter方法设置y坐标
public void setY(double y) {
this.y = y;
}
@Override
public String toString() {
return "Point{" +
"x=" + x +
", y=" + y +
'}';
}
public static void main(String[] args) {
// 创建坐标原点
Point origin = new Point();
System.out.println("Origin: " + origin);
// 创建任意点
Point arbitraryPoint = new Point(3.5, 4.7);
System.out.println("Arbitrary Point: " + arbitraryPoint);
}
}
```
在这个`Point`类中,我们定义了初始化坐标、读取和设置坐标值的方法,并实现了`toString()`方法以便于打印。`main`函数则展示了如何创建并操作坐标点。
编写Java程序,设计Circle类和Point类,Point类表示平面直角坐标系中的几何点,具有横坐标x和纵坐标y两个属性;Circle类具有以下属性和方法: A.center-圆心 B.radius-半径 对属性进行封装;--- 构造方法 A.无参构造方法:对内部字段进行初始化 B.带有两个参数的构造方法 实例方法: A.getLength():求圆周长 B.getArea(): 求圆面积 静态方法: A.distance(Circle c1,Circle c2): 求两个圆的距离 isCrossed(Circle c1,Circle c2): 判断两个圆是否相交
Point类代码如下:
```java
public class Point {
private double x;
private double y;
public Point() {
this(0, 0);
}
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
}
```
Circle类代码如下:
```java
public class Circle {
private Point center;
private double radius;
public Circle() {
this(new Point(), 0);
}
public Circle(Point center, double radius) {
this.center = center;
this.radius = radius;
}
public Point getCenter() {
return center;
}
public void setCenter(Point center) {
this.center = center;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getLength() {
return 2 * Math.PI * radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public static double distance(Circle c1, Circle c2) {
double dx = c1.getCenter().getX() - c2.getCenter().getX();
double dy = c1.getCenter().getY() - c2.getCenter().getY();
double distance = Math.sqrt(dx * dx + dy * dy);
return distance - c1.getRadius() - c2.getRadius();
}
public static boolean isCrossed(Circle c1, Circle c2) {
double distance = distance(c1, c2);
if (distance < 0) {
return true;
} else {
return false;
}
}
}
```
其中,distance方法用勾股定理求出两个圆心的距离,然后减去两个圆的半径和,即为两个圆的距离。isCrossed方法判断两个圆的距离是否小于0,如果小于0,则说明两个圆相交。
阅读全文
相关推荐
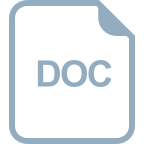
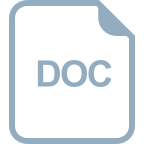
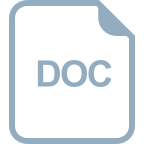










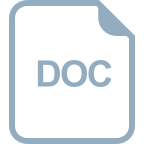
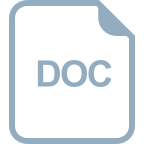
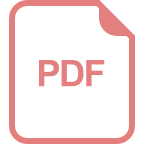
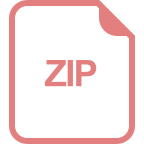