devc++mysql
时间: 2023-09-26 20:08:37 浏览: 105
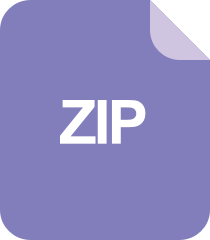
devC++开发的连接mysql
MySQL is an open-source relational database management system. It is one of the most popular relational database management systems and is widely used in web applications. MySQL is written in C and C++ and is developed by Oracle Corporation. It is used to store and retrieve data for websites, applications, and other software. MySQL is known for its speed and scalability, making it ideal for large-scale web applications. It is also known for its ease of use and its support for a variety of programming languages. MySQL is free and open-source software, which means that it can be used, modified, and distributed without any licensing fees.
阅读全文
相关推荐
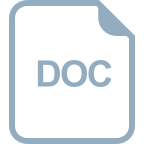
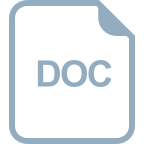
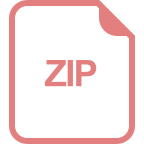


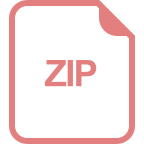
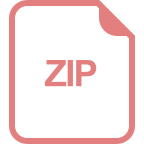
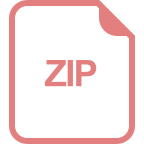








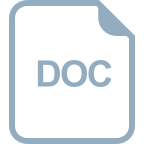