Linux进程练习 1、参考课件中的方法,用fork创建新进程,并观察父子进程之间的运行差异。 2、参考课件中介绍的方法,创建Linux的多线程程序。 其他要求: 1、用C或C++实现,命令行编译。 2、至少使用两个C或C++文件,不得在一个文件内书写所有代码。(学会用makefile模式管理多个C文件的编译方式。) 以上内容要求有关键的截图和必要的文字说明。
时间: 2023-03-24 13:04:17 浏览: 110
关于Linux进程练习的问题,我可以回答。
1、使用fork创建新进程,观察父子进程之间的运行差异。
首先,我们需要在命令行中编写C或C++代码,使用fork函数创建新进程。在父进程和子进程中,我们可以使用不同的代码来观察它们之间的运行差异。
下面是一个示例代码:
```
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t pid;
int count = ;
pid = fork();
if (pid < ) {
printf("Error: fork failed.\n");
return 1;
} else if (pid == ) {
printf("Child process: pid=%d\n", getpid());
while (count < 5) {
printf("Child process: count=%d\n", count);
count++;
sleep(1);
}
} else {
printf("Parent process: pid=%d, child pid=%d\n", getpid(), pid);
while (count < 5) {
printf("Parent process: count=%d\n", count);
count++;
sleep(1);
}
}
return ;
}
```
在这个示例代码中,我们使用了fork函数创建了一个新的进程。在子进程中,我们使用了while循环来输出一些信息,并使用sleep函数来暂停进程的执行。在父进程中,我们也使用了类似的代码来输出信息。
运行这个程序后,我们可以看到父子进程之间的运行差异。子进程会先输出一些信息,然后父进程才会输出信息。这是因为子进程和父进程是并发执行的,它们之间的运行顺序是不确定的。
2、创建Linux的多线程程序。
要创建Linux的多线程程序,我们可以使用pthread库。下面是一个示例代码:
```
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg) {
int i;
for (i = ; i < 5; i++) {
printf("Thread: %d\n", i);
sleep(1);
}
return NULL;
}
int main() {
pthread_t tid;
int ret;
ret = pthread_create(&tid, NULL, thread_func, NULL);
if (ret != ) {
printf("Error: pthread_create failed.\n");
return 1;
}
int i;
for (i = ; i < 5; i++) {
printf("Main thread: %d\n", i);
sleep(1);
}
pthread_join(tid, NULL);
return ;
}
```
在这个示例代码中,我们使用了pthread库来创建一个新的线程。在新线程中,我们使用了for循环来输出一些信息,并使用sleep函数来暂停线程的执行。在主线程中,我们也使用了类似的代码来输出信息。
运行这个程序后,我们可以看到新线程和主线程之间的运行差异。新线程会先输出一些信息,然后主线程才会输出信息。这是因为新线程和主线程是并发执行的,它们之间的运行顺序是不确定的。
以上就是关于Linux进程练习的回答,希望能对您有所帮助。
阅读全文
相关推荐
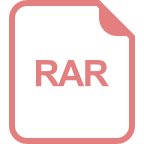

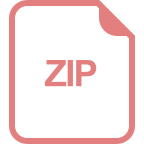
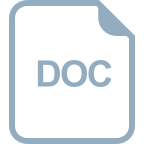
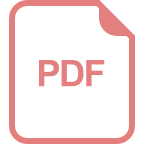
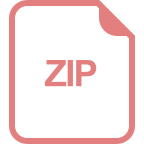
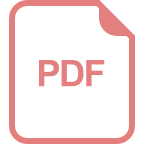
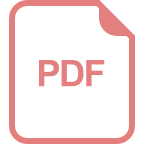
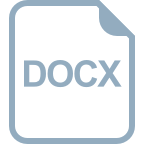
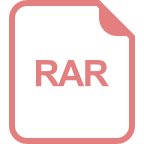
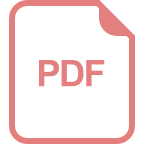
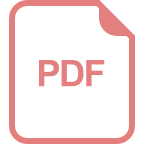
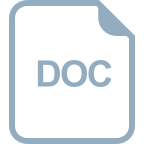
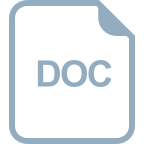
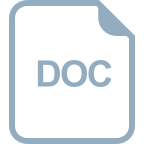
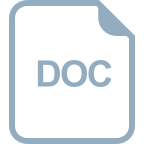
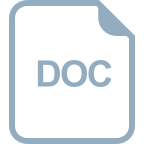