如何用python和opencv在图片中自动定位A4纸并裁剪出来
时间: 2023-06-16 21:05:07 浏览: 112
首先,你需要安装OpenCV库。以下是一个步骤:
1. 安装OpenCV:
```python
!pip install opencv-python
```
2. 导入OpenCV库:
```python
import cv2
```
3. 读取图片文件:
```python
img = cv2.imread('image.jpg')
```
4. 转换为灰度图像:
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
5. 进行图像平滑处理,以便更好地定位边缘(可以尝试不同的核大小):
```python
blur = cv2.GaussianBlur(gray, (5, 5), 0)
```
6. 检测边缘:
```python
edges = cv2.Canny(blur, 100, 200)
```
7. 查找轮廓:
```python
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
8. 遍历轮廓,找到最大的四边形:
```python
max_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_area:
max_area = area
max_contour = contour
approx = cv2.approxPolyDP(max_contour, 0.01 * cv2.arcLength(max_contour, True), True)
if len(approx) == 4:
cv2.drawContours(img, [approx], -1, (0, 255, 0), 2)
```
9. 裁剪出A4纸:
```python
width = 210
height = 297
pts1 = np.float32([approx[0], approx[1], approx[2], approx[3]])
pts2 = np.float32([[0, 0], [width, 0], [width, height], [0, height]])
matrix = cv2.getPerspectiveTransform(pts1, pts2)
result = cv2.warpPerspective(img, matrix, (width, height))
```
完整代码如下:
```python
import cv2
import numpy as np
# 读取图片文件
img = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行图像平滑处理
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 检测边缘
edges = cv2.Canny(blur, 100, 200)
# 查找轮廓
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓,找到最大的四边形
max_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_area:
max_area = area
max_contour = contour
approx = cv2.approxPolyDP(max_contour, 0.01 * cv2.arcLength(max_contour, True), True)
if len(approx) == 4:
cv2.drawContours(img, [approx], -1, (0, 255, 0), 2)
# 裁剪出A4纸
width = 210
height = 297
pts1 = np.float32([approx[0], approx[1], approx[2], approx[3]])
pts2 = np.float32([[0, 0], [width, 0], [width, height], [0, height]])
matrix = cv2.getPerspectiveTransform(pts1, pts2)
result = cv2.warpPerspective(img, matrix, (width, height))
# 显示结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:这个方法并不保证一定能够正确地识别出A4纸,因此需要对代码进行调整和优化,以便适应不同的图片。
阅读全文
相关推荐
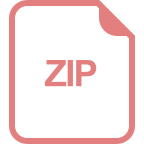
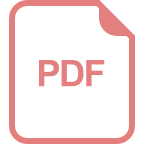
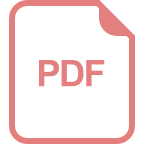















