ASP.NET Core 7项目启动的时候自动启动 十个定时任务 不影响主线程的http请求 每个的周期不一样
时间: 2024-04-13 16:30:56 浏览: 12
要在ASP.NET Core 7项目启动时自动启动十个定时任务,并且每个任务的周期不同,并且不影响主线程的HTTP请求,可以使用`System.Threading.Timer`来执行这些定时任务。`System.Threading.Timer`是一个定时器类,它可以在指定的时间间隔内重复执行指定的回调方法。下面是一个示例代码:
```csharp
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Threading;
using System.Threading.Tasks;
public class MyTimedService : IHostedService, IDisposable
{
private Dictionary<int, int> _taskIntervals = new Dictionary<int, int>
{
{ 0, 1000 }, // 任务0的周期为1秒
{ 1, 2000 }, // 任务1的周期为2秒
// 其他任务的周期设置
};
private List<Timer> _timers = new List<Timer>();
public Task StartAsync(CancellationToken cancellationToken)
{
foreach (var taskInterval in _taskIntervals)
{
int taskId = taskInterval.Key;
int interval = taskInterval.Value;
Timer timer = new Timer(async (_) => await DoWork(taskId), null, TimeSpan.Zero, TimeSpan.FromMilliseconds(interval));
_timers.Add(timer);
}
return Task.CompletedTask;
}
private async Task DoWork(int taskId)
{
// 执行定时任务的逻辑
// 使用taskId来区分不同的任务
await Task.Delay(100); // 模拟任务执行时间
}
public async Task StopAsync(CancellationToken cancellationToken)
{
foreach (var timer in _timers)
{
timer.Dispose();
}
await Task.CompletedTask;
}
public void Dispose()
{
foreach (var timer in _timers)
{
timer.Dispose();
}
}
}
```
在此示例中,我们使用`System.Threading.Timer`类来创建定时器,并为每个任务设置不同的周期。我们使用`Timer`构造函数的参数来指定定时器的回调方法,并使用`TimeSpan.Zero`和`TimeSpan.FromMilliseconds`来设置定时器的初始延迟和重复间隔。在回调方法中执行定时任务的逻辑。
请注意,在`ConfigureServices`方法中注册`MyTimedService`时仍然使用`AddSingleton`方法。
```csharp
using Microsoft.Extensions.DependencyInjection;
public void ConfigureServices(IServiceCollection services)
{
// 省略其他代码...
services.AddSingleton<MyTimedService>();
// 省略其他代码...
}
```
通过这种方式,您可以在ASP.NET Core 7项目启动时自动启动十个定时任务,并且每个任务的周期不同,同时不影响主线程的HTTP请求。
相关推荐
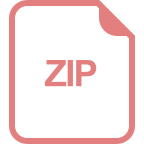
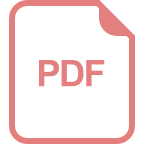
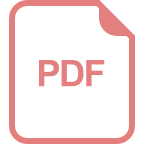
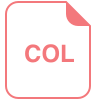
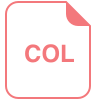
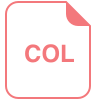
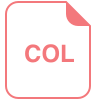
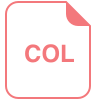









