html烟花代码在线编程
时间: 2024-01-02 20:03:23 浏览: 30
以下是一个使用HTML5 Canvas和JavaScript创建烟花特效的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>烟花特效</title>
<style>
canvas {
background-color: black;
}
</style>
</head>
<body>
<canvas id="fireworksCanvas"></canvas>
<script>
// 获取画布元素
var canvas = document.getElementById("fireworksCanvas");
var ctx = canvas.getContext("2d");
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 创建烟花对象
function Firework() {
this.x = Math.random() * canvas.width; this.y = canvas.height;
this.vx = Math.random() * 2 - 1;
this.vy = Math.random() * -10 - 10;
this.gravity = 0.2;
this.opacity = 1;
this.color = getRandomColor();
this.update = function() {
this.x += this.vx;
this.y += this.vy;
this.vy += this.gravity;
this.opacity -= 0.01;
// 绘制烟花
ctx.beginPath();
ctx.arc(this.x, this.y, 3, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.opacity; ctx.fill();
}
}
// 生成随机颜色
function getRandomColor() {
var letters = "0123456789ABCDEF";
var color = "#";
for (var i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
// 创建烟花数组
var fireworks = [];
// 更新画布
function updateCanvas() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 更新烟花数组
for (var i = 0; i < fireworks.length; i++) {
fireworks[i].update();
if (fireworks[i].opacity <= 0) {
fireworks.splice(i, 1);
}
}
// 添加新的烟花
if (Math.random() < 0.05) {
fireworks.push(new Firework());
}
// 递归调用更新画布
requestAnimationFrame(updateCanvas);
}
// 启动动画
updateCanvas();
</script>
</body>
</html>
```
你可以将上述代码复制到一个HTML文件中,并在浏览器中打开该文件,即可看到烟花特效。
相关推荐
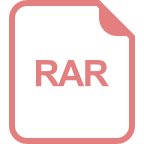
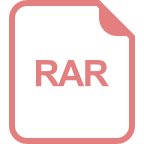
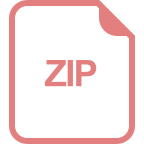










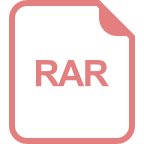
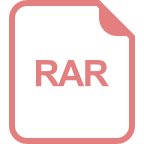
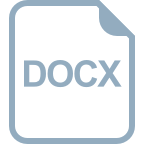