给我遗传算法的matlab构建框架
时间: 2024-09-30 22:09:24 浏览: 29
遗传算法(Genetic Algorithm, GA)是一种模拟自然选择过程的优化搜索算法,常用于解决复杂的优化问题。在MATLAB中构建一个基本的遗传算法框架,通常包括以下几个步骤:
1. **初始化种群**:创建一组随机生成的个体作为初始种群,每个个体是一个包含解向量的结构或向量。
```matlab
function population = initializePopulation(size, problem)
population = cell(size, 1);
for i = 1:size
population{i} = generateIndividual(problem.lowerBound, problem.upperBound); % 根据问题范围生成随机个体
end
end
```
2. **适应度函数**:定义衡量个体优劣的函数,比如目标函数值。
```matlab
function fitness = evaluateFitness(individual, problem)
fitness = objectiveFunction(individual, problem); % 调用具体的优化函数计算适应度
end
```
3. **选择操作**:从当前种群中按适应度概率选择下一代个体。
```matlab
function selectedParents = selection(population, fitness, ratio)
[~, parents] = sort(fitness, 'descend'); % 按适应度降序排序
selectedParents = parents(1:round(ratio * length(parents)));
end
```
4. **交叉操作**:通过两个或更多父母个体产生新的后代。
```matlab
function children = crossover(parents, crossoverRate, problem)
numChildren = round(length(parents) * crossoverRate);
children = cell(numChildren, 1);
for i = 1:numChildren
parentPair = chooseRandomPair(parents);
children{i} = crossoverOperator(parentPair, problem);
end
end
```
5. **变异操作**:对新产生的后代进行微小改变,增加多样性。
```matlab
function mutatedChild = mutation(child, mutationRate, problem)
if rand < mutationRate
child = mutateOperator(child, problem);
end
end
```
6. **更新种群**:将新生成的个体添加到种群并淘汰最差的部分。
```matlab
function newPopulation = updatePopulation(currentPopulation, selectedParents, mutatedChildren, problem)
newPopulation = [selectedParents; mutatedChildren];
while length(newPopulation) > problem.populationSize
worstIndividual = min(newPopulation, 'fitness');
newPopulation = setdiff(newPopulation, worstIndividual);
end
end
% 主循环
while not(converged || maxIterationsReached)
currentPopulation = ... % 更新种群
% 计算适应度
fitnesses = arrayfun(@(x) evaluateFitness(x, problem), currentPopulation);
... % 进行选择、交叉、变异等操作
end
```
在实际应用中,这只是一个基础框架,你可以根据需要调整参数、改进选择策略、交叉和变异操作等。最后的`converged`和`maxIterationsReached`条件用于控制算法的终止条件。
阅读全文
相关推荐
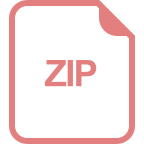
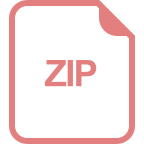
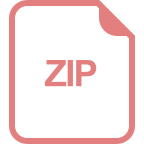















