编一个程序,测试字符串s1中第一次出现在字符串s2中的字符的位置。要求定义一个函数,返回出现这个字符的位置;若s1中不含有s2的字符,则返回-1,用C语言
时间: 2024-09-07 14:04:09 浏览: 62
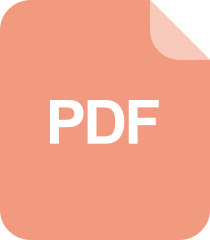
PHP中一个控制字符串输出的函数
要完成这个任务,我们可以通过遍历字符串s1,检查每一个字符是否出现在字符串s2中。当找到第一个出现在s2中的字符时,记录它的位置并返回。如果没有找到这样的字符,则返回-1。以下是一个C语言的函数实现,该函数接受两个字符串s1和s2作为参数,并返回上述要求的结果:
```c
#include <stdio.h>
// 函数声明
int findFirstOccurrence(const char *s1, const char *s2);
int main() {
const char *s1 = "Hello World";
const char *s2 = "lo";
int position = findFirstOccurrence(s1, s2);
printf("在字符串\"%s\"中,字符串\"%s\"第一次出现的字符位置是: %d\n", s1, s2, position);
return 0;
}
// 函数定义
int findFirstOccurrence(const char *s1, const char *s2) {
const char *p;
while (*s1 != '\0') { // 遍历字符串s1
p = s2;
while (*p != '\0' && *p != *s1) { // 遍历字符串s2
++p;
}
if (*p != '\0') { // 如果在s2中找到了与s1当前位置相同的字符
return s1 - s2; // 返回位置索引
}
++s1;
}
return -1; // 如果没有找到,则返回-1
}
```
在这段代码中,`findFirstOccurrence`函数通过两个嵌套循环来实现查找。外层循环遍历`s1`中的每个字符,内层循环则检查当前字符是否存在于`s2`中。如果存在,返回当前字符在`s1`中的位置;如果遍历完整个`s1`都没有找到,则返回-1。
阅读全文
相关推荐
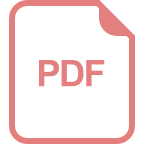
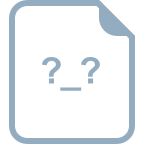















