用c++编写一个程序,将一个子字符串s2插入到主字符串s1中,其起始插入位置为n
时间: 2024-02-21 09:58:09 浏览: 74
好的,下面是用 C++ 编写的程序,实现将一个子字符串 s2 插入到主字符串 s1 中,其起始插入位置为 n:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string s1, s2;
int n;
cout << "请输入主字符串 s1:";
cin >> s1;
cout << "请输入子字符串 s2:";
cin >> s2;
cout << "请输入起始插入位置 n:";
cin >> n;
s1.insert(n, s2);
cout << "插入后的字符串为:" << s1 << endl;
return 0;
}
```
程序运行示例:
```
请输入主字符串 s1:hello world
请输入子字符串 s2:, my name is John
请输入起始插入位置 n:6
插入后的字符串为:hello , my name is Johnworld
```
注意:该程序只是简单地演示了如何使用 C++ 的 string 类型的 insert() 函数实现字符串插入操作,实际应用中还需要考虑更多的细节问题,比如输入的字符串长度、起始插入位置是否合法等等。
相关问题
请使用C++编写一个程序,将一个子字符s2插入到主字符s1中,其起始插入位置为n
以下是一个使用C++标准库的示例程序,可以将字符串s2插入到字符串s1的第n个位置:
```
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s1 = "Hello, world!";
string s2 = "beautiful ";
int n = 7;
s1.insert(n, s2);
cout << s1 << endl;
return 0;
}
```
在这个程序中,我们首先定义了两个字符串变量s1和s2,以及一个整型变量n,用于指定插入位置。然后,我们使用string类的insert方法将s2插入到s1的第n个位置。最后,我们输出修改后的s1字符串。
如果需要手动实现字符串插入功能,可以使用C++标准库中的字符串操作函数,例如strcpy、strcat和strlen等。以下是一个使用这些函数手动实现字符串插入的示例程序:
```
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
char s1[] = "Hello, world!";
char s2[] = "beautiful ";
int n = 7;
int len1 = strlen(s1);
int len2 = strlen(s2);
// 将s1的第n个位置及之后的字符向后移动len2个位置
for (int i = len1; i >= n; i--)
{
s1[i + len2] = s1[i];
}
// 将s2插入到s1的第n个位置
for (int i = 0; i < len2; i++)
{
s1[n + i] = s2[i];
}
cout << s1 << endl;
return 0;
}
```
在这个程序中,我们首先定义了两个字符数组s1和s2,以及一个整型变量n,用于指定插入位置。然后,我们使用strlen函数获取s1和s2的长度。接着,我们通过循环将s1的第n个位置及之后的字符向后移动len2个位置,为插入s2腾出空间。最后,我们再循环将s2插入到s1的第n个位置。最终,我们输出修改后的s1字符串。
编写一个程序,输入两个字符串 s1 和 s2 ,判断 s2 是否是 s1 的子串,如果是,则输出 s2 在 s1 中的起始位置。否则输出 0 输入:两个字符串 s1 和 s2 输出:s2 在 s1 中的起始位置 或者 输出 0
好的,以下是用 C++ 实现的程序:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int kmp(const string& s1, const string& s2) {
int n1 = s1.size(), n2 = s2.size();
vector<int> nxt(n2);
int i = 0, j = -1;
// 构建 next 数组
nxt[0] = -1;
while (i < n2 - 1) {
if (j == -1 || s2[i] == s2[j]) {
i++, j++;
nxt[i] = (s2[i] == s2[j]) ? nxt[j] : j;
} else {
j = nxt[j];
}
}
// 匹配
i = 0, j = 0;
while (i < n1 && j < n2) {
if (j == -1 || s1[i] == s2[j]) {
i++, j++;
} else {
j = nxt[j];
}
}
if (j == n2) {
return i - n2;
} else {
return 0;
}
}
int main() {
string s1, s2;
int pos;
cout << "请输入字符串 s1 和 s2:" << endl;
cin >> s1 >> s2;
pos = kmp(s1, s2);
if (pos) {
cout << "s2 在 s1 中的起始位置是:" << pos << endl;
} else {
cout << "s2 不是 s1 的子串。" << endl;
}
return 0;
}
```
这个程序与 C 语言版本类似,使用了 C++ 的 STL 库来简化字符串处理。主要的算法部分是 `kmp` 函数,该函数同样实现了 KMP 算法,用于在 `s1` 中匹配 `s2` 是否为其子串。在 `main` 函数中,读入两个字符串并调用 `kmp` 函数进行匹配,输出匹配结果。
阅读全文
相关推荐
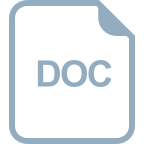
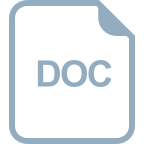
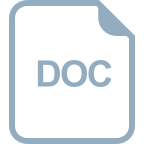



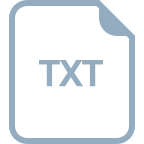









