输入两个字符串,验证其中一个串是否为另一个串的子串。C++
时间: 2024-02-28 08:53:36 浏览: 143
可以使用C++中的string的find函数来判断一个字符串是否为另一个字符串的子串。具体实现如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string s1, s2;
cin >> s1 >> s2;
if (s1.find(s2) != string::npos) {
cout << "Yes" << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
其中,find函数返回一个字符串中是否找到了另一个字符串的位置,如果找到了则返回该字符串的起始位置,否则返回string::npos。如果返回的不是npos,则说明s2是s1的子串。
相关问题
输入两个字符串s1和$2。验证其中一个字符串是否是另一个字符串的子串。用c++
在C++中,验证一个字符串`s1`是否是另一个字符串`s2`的子串,可以使用`substr()`函数或者直接遍历的方式。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
bool isSubstr(const std::string &s1, const std::string &s2) {
// 使用substr()函数检查s1是否是s2的子串
if (s2.find(s1) != std::string::npos) {
return true;
} else {
return false;
}
}
int main() {
std::string s1 = "hello";
std::string s2 = "world hello";
if (isSubstr(s1, s2)) {
std::cout << s1 << " is a substring of " << s2 << std::endl;
} else {
std::cout << s1 << " is not a substring of " << s2 << std::endl;
}
return 0;
}
```
在这个例子中,`find()`函数用于查找`s1`在`s2`中的位置。如果找到(返回值不是`std::string::npos`),则说明`s1`是`s2`的子串。
用C++编写一段程序:输入两个字符串,验证其中一个串是否为另一个的字串。输入:输入两个字符串,每个字符串占一行,长度不超过200且不含空格。输出:若第一个串s1是第二个串s2的子串,输出(s1)is substring of (s2),否则,若第二个串s2是第一个串s1的子串,输出(s2) is substring of (s1),否则,输出 No substring
下面是用C++编写的程序,用于验证一个字符串是否为另一个字符串的子串。
```cpp
#include <iostream>
#include <string>
int main() {
std::string s1, s2;
std::getline(std::cin, s1);
std::getline(std::cin, s2);
if (s1.find(s2) != std::string::npos) {
std::cout << s2 << " is substring of " << s1 << std::endl;
} else if (s2.find(s1) != std::string::npos) {
std::cout << s1 << " is substring of " << s2 << std::endl;
} else {
std::cout << "No substring" << std::endl;
}
return 0;
}
```
请将两个字符串分别输入到程序的标准输入中的两行,然后程序将输出结果。如果第一个字符串是第二个字符串的子串,将输出 "(s1) is substring of (s2)";如果第二个字符串是第一个字符串的子串,将输出 "(s2) is substring of (s1)";如果两个字符串没有子串关系,将输出 "No substring"。
阅读全文
相关推荐
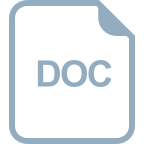
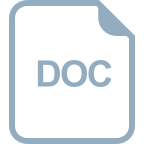
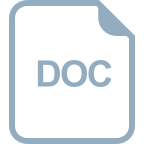





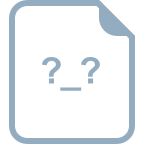
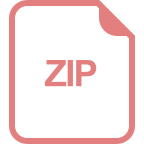
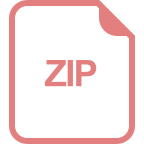
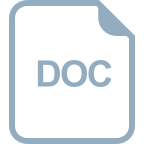
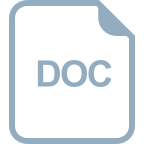
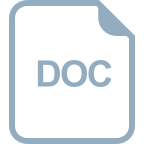

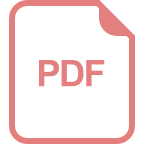