struct book library;//把library设为一个可以使用book结构体的结构体变量,则library这个变量就包含了其book结构体中的所有元素
时间: 2024-09-10 13:06:28 浏览: 33
在C语言中,`struct book library;` 定义了一个名为 `library` 的结构体变量,该结构体是由 `book` 结构体派生的。这意味着 `library` 变量将拥有 `book` 结构体的所有成员变量。`book` 结构体可能包含作者、书名、ISBN等属性,而 `library` 则可以用作存放书籍信息的容器,比如图书馆的藏书记录。
例如,如果你有这样一个 `book` 结构体:
```c
struct book {
char author[50];
char title[100];
int isbn;
};
```
那么 `library` 结构体将会是这样的:
```c
struct book library;
```
你可以创建 `library` 的实例,并通过它访问和操作 `book` 中的数据:
```c
void main() {
struct book myBook = {"John Doe", "The Great Adventure", 1234567890};
// 现在 library 就可以存储 myBook 的内容
library = myBook;
// 访问 library 的数据
printf("Author: %s\n", library.author);
printf("Title: %s\n", library.title);
printf("ISBN: %d\n", library.isbn);
}
```
相关问题
定义一个图书类的结构体(成员有书名 编号和价格)变量为4 通过数据块函数把4个变量的内容写入文件中,然后读取文件内容打印到屏幕上
好的,这是一个C++的图书类结构体的定义,包括数据块写入文件和读取文件内容的操作:
```c++
#include <iostream>
#include <fstream> // 包含文件读写相关的头文件
using namespace std;
// 图书类结构体
struct Book {
string title; // 书名
int id; // 编号
double price; // 价格
};
int main() {
// 定义4本书
Book books[4] = {
{"C++ Primer", 101, 59.9},
{"Effective C++", 102, 39.8},
{"The C++ Standard Library", 103, 78.9},
{"Thinking in C++", 104, 29.9}
};
// 将4本书的内容写入文件中
ofstream outfile("book.txt", ios::binary); // 打开文件
if (outfile.is_open()) {
outfile.write(reinterpret_cast<char*>(&books), sizeof(books));
outfile.close(); // 关闭文件
}
else {
cout << "Error opening file!" << endl;
return -1;
}
// 从文件中读取内容并打印到屏幕上
ifstream infile("book.txt", ios::binary); // 打开文件
if (infile.is_open()) {
Book books_read[4];
infile.read(reinterpret_cast<char*>(&books_read), sizeof(books_read));
infile.close(); // 关闭文件
for (int i = 0; i < 4; i++) {
cout << "Book " << i+1 << ": " << endl;
cout << "Title: " << books_read[i].title << endl;
cout << "ID: " << books_read[i].id << endl;
cout << "Price: " << books_read[i].price << endl;
cout << endl;
}
}
else {
cout << "Error opening file!" << endl;
return -1;
}
return 0;
}
```
运行这段代码后,会在当前目录下生成一个名为`book.txt`的文件,里面包含了4本书的信息。接着,程序会从该文件中读取信息并打印到屏幕上,输出结果如下:
```
Book 1:
Title: C++ Primer
ID: 101
Price: 59.9
Book 2:
Title: Effective C++
ID: 102
Price: 39.8
Book 3:
Title: The C++ Standard Library
ID: 103
Price: 78.9
Book 4:
Title: Thinking in C++
ID: 104
Price: 29.9
```
用C语言编写一个结构体,要求如下:设计一个图书馆信息结构体,包括有,书号,书名,作者,出版社,出版日期,
以下是一个示例代码,实现了题目要求的结构体:
```c
#include <stdio.h>
#include <string.h>
// 定义图书馆信息结构体
struct Library {
char book_number[20];
char book_name[50];
char author[30];
char publisher[50];
char publish_date[20];
};
int main() {
// 定义一个图书馆信息结构体变量
struct Library book;
// 输入图书信息
printf("请输入书号:");
scanf("%s", book.book_number);
printf("请输入书名:");
scanf("%s", book.book_name);
printf("请输入作者:");
scanf("%s", book.author);
printf("请输入出版社:");
scanf("%s", book.publisher);
printf("请输入出版日期:");
scanf("%s", book.publish_date);
// 输出图书信息
printf("\n图书信息如下:\n");
printf("书号:%s\n", book.book_number);
printf("书名:%s\n", book.book_name);
printf("作者:%s\n", book.author);
printf("出版社:%s\n", book.publisher);
printf("出版日期:%s\n", book.publish_date);
return 0;
}
```
在上述代码中,我们使用了 `struct Library` 来定义了一个图书馆信息的结构体,包括了书号、书名、作者、出版社和出版日期这些字段。在 `main` 函数中,我们定义了一个 `struct Library` 类型的变量 `book`,并通过 `scanf` 函数输入了图书信息。最后,我们使用 `printf` 函数输出了图书信息。
阅读全文
相关推荐
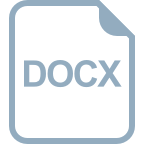
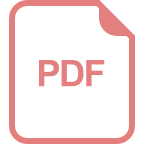
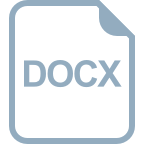
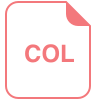
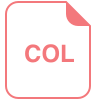
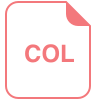










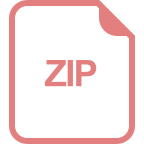
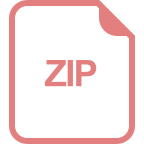