HttpURLConnection 单向https认证
时间: 2023-08-26 16:03:06 浏览: 129
在使用 `HttpURLConnection` 进行单向HTTPS认证时,可以通过以下步骤实现:
1. 创建一个信任管理器:
```java
TrustManager[] trustAllCerts = new TrustManager[] {
new X509TrustManager() {
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
// 不验证客户端证书
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
// 验证服务器证书
try {
chain[0].checkValidity();
} catch (Exception e) {
throw new CertificateException("证书无效");
}
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
}
};
```
2. 创建一个SSL上下文:
```java
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, trustAllCerts, new SecureRandom());
```
3. 设置`HttpURLConnection`的SSL Socket Factory:
```java
HttpsURLConnection.setDefaultSSLSocketFactory(sslContext.getSocketFactory());
```
4. 发起HTTPS请求:
```java
URL url = new URL("https://example.com");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 可选:设置其他请求参数
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
// 发起请求
int responseCode = connection.getResponseCode();
// 处理响应
if (responseCode == HttpURLConnection.HTTP_OK) {
// 读取响应数据
InputStream inputStream = connection.getInputStream();
// ...
} else {
// 处理错误情况
}
```
以上代码将忽略对客户端证书的验证,只验证服务器证书的有效性。同样需要注意,在生产环境中建议使用双向HTTPS认证来增强安全性,即同时验证客户端和服务器证书。
阅读全文
相关推荐
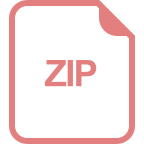
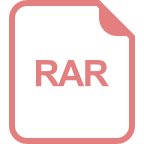

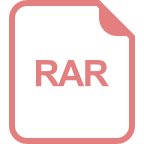
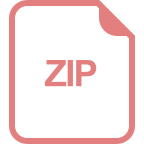
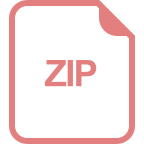
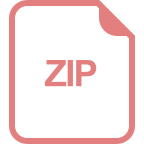
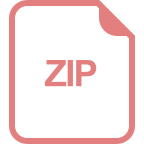
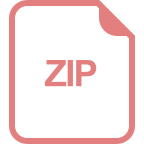
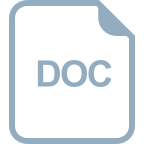




