利用c++添加复数的加减
时间: 2024-09-14 12:04:07 浏览: 42
在C++中添加和减去复数可以通过重载加号(+)和减号(-)运算符来实现。首先,需要创建一个复数类(Complex),然后在这个类中重载这两个运算符。以下是实现复数加减的一个简单示例:
```cpp
#include <iostream>
class Complex {
public:
double real; // 复数的实部
double imag; // 复数的虚部
// 构造函数
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
// 加法运算符重载
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法运算符重载
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 输出函数
void print() const {
if (imag < 0)
std::cout << real << " - " << -imag << "i\n";
else
std::cout << real << " + " << imag << "i\n";
}
};
int main() {
Complex c1(4.0, 3.0); // 实例化两个复数对象
Complex c2(2.0, -1.0);
Complex sum = c1 + c2; // 复数加法
Complex diff = c1 - c2; // 复数减法
std::cout << "Sum of c1 and c2: ";
sum.print(); // 输出结果
std::cout << "Difference of c1 and c2: ";
diff.print(); // 输出结果
return 0;
}
```
在上述代码中,`Complex`类代表复数,其中包含实部`real`和虚部`imag`。我们重载了加号和减号运算符,使得可以使用这些运算符来对复数进行加减运算。运算符重载的函数返回一个新的`Complex`对象,该对象代表了运算的结果。
阅读全文
相关推荐
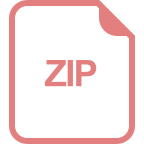
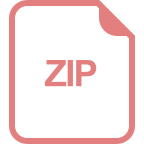
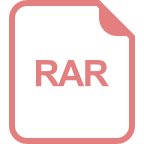
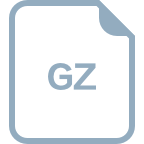
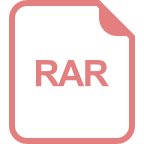
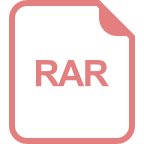
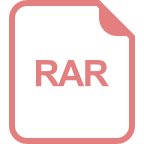
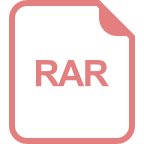
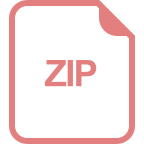
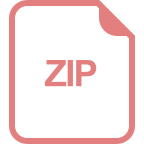
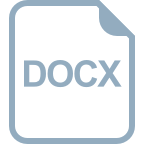
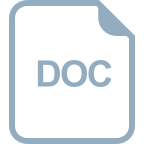
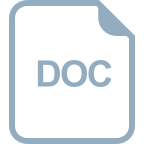
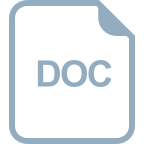
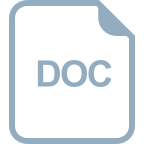
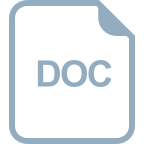
