使用【c++】写本实验利用C++面向对象的编程知识设计一个特殊的计算器,可以实现复数、有理数、矩阵的特殊计算。其中复数运算实现复数的加法、减法、乘法和除法的基本功能;矩阵运算实现矩阵的加法、减法和乘法的基本功能;有理数运算实现有理数的加法、减法和乘法的基本功能。代码
时间: 2024-12-12 19:27:06 浏览: 5
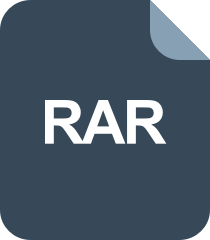
Billiards.rar_C++_Builder_

在C++中设计这样一个特殊的计算器,首先需要创建三个类分别表示复数、有理数和矩阵,并提供相应的操作函数。这里是一个简化的实现示例:
```cpp
#include <iostream>
#include <vector>
// 定义复数类 Complex
class Complex {
public:
double real;
double imag;
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 加法
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 乘法
Complex operator*(const Complex& other) const {
double new_real = real * other.real - imag * other.imag;
double new_imag = real * other.imag + imag * other.real;
return Complex(new_real, new_imag);
}
// 除法,注意除以零的情况
Complex operator/(const Complex& other) const {
if (other.imag == 0 && other.real != 0)
throw std::runtime_error("Division by zero is not allowed");
double denominator = other.real * other.real + other.imag * other.imag;
double new_real = (real * other.real + imag * other.imag) / denominator;
double new_imag = (imag * other.real - real * other.imag) / denominator;
return Complex(new_real, new_imag);
}
};
// 有理数类 Rational
class Rational {
private:
int numerator;
int denominator;
public:
// 构造函数
Rational(int num = 0, int den = 1) : numerator(num), denominator(den) {}
// ... 类似复数的加、减、乘函数
// 乘法,保持分母不变
Rational operator*(const Rational& other) const {
int new_num = numerator * other.numerator;
return Rational(new_num, denominator);
}
// 其他操作同理
};
// 矩阵类 Matrix
class Matrix {
private:
std::vector<std::vector<int>> data;
public:
// 构造函数
Matrix(std::vector<int> row_data) : data(row_data) {}
// 加法和减法
Matrix operator+(const Matrix& other) const {
Matrix result(data.size(), data[0].size());
for (int i = 0; i < data.size(); ++i) {
for (int j = 0; j < data[i].size(); ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 乘法,假设输入矩阵是方阵
Matrix operator*(const Matrix& other) const {
Matrix result(other.columns(), data.size());
for (int i = 0; i < result.rows(); ++i) {
for (int j = 0; j < other.columns(); ++j) {
int sum = 0;
for (int k = 0; k < data.columns(); ++k) {
sum += data[i][k] * other.data[k][j];
}
result.data[i][j] = sum;
}
}
return result;
}
};
// 示例如何使用
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
std::cout << "Complex addition: " << c1 + c2 << std::endl;
Rational r1(2, 3);
Rational r2(4, 5);
std::cout << "Rational multiplication: " << r1 * r2 << std::endl;
Matrix m1({1, 2, 3}, {4, 5, 6});
Matrix m2({7, 8, 9}, {10, 11, 12});
std::cout << "Matrix addition: " << m1 + m2 << std::endl;
return 0;
}
```
这个示例展示了基本的复数、有理数和矩阵类的设计以及一些基本操作。实际项目中,你需要添加更多的错误处理和验证功能。
阅读全文
相关推荐
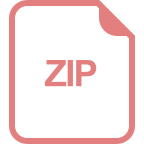
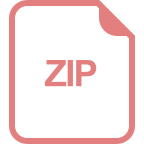
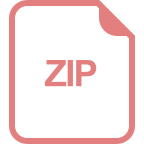
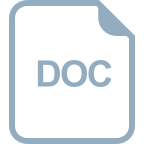














