在Linux下用C获取电脑可用wifi的代码
时间: 2023-08-31 16:19:03 浏览: 113
### 回答1:
获取电脑可用wifi的代码可以使用Linux下的命令行工具iwlist。
下面是使用C语言调用iwlist命令获取可用wifi列表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BUF_SIZE 1024
int main() {
FILE *fp;
char buffer[MAX_BUF_SIZE];
char cmd[] = "iwlist scan | grep 'ESSID' | awk '{print $NF}' | sed 's/\\\"//g'";
fp = popen(cmd, "r");
if (fp == NULL) {
printf("Failed to run command\n" );
exit(1);
}
while (fgets(buffer, MAX_BUF_SIZE, fp) != NULL) {
buffer[strcspn(buffer, "\n")] = 0;
printf("%s\n", buffer);
}
pclose(fp);
return 0;
}
```
这个程序调用了Linux下的命令行工具iwlist来扫描可用的wifi列表,然后使用grep、awk和sed命令进行过滤和格式化输出。通过调用popen()函数来执行命令,并通过fgets()函数逐行读取输出结果。
输出的结果是一个wifi名称的列表,每个名称占一行,可以进一步处理或显示给用户。
### 回答2:
获取Linux电脑可用WiFi的代码可以通过使用C语言和Linux系统提供的API来实现。首先,需要包含相关的头文件。然后,使用SIOCGIFCONF命令获取网络接口列表,并遍历列表找到无线网络接口。
以下是一个示例代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <sys/socket.h>
#include <net/if.h>
#include <netinet/in.h>
#include <arpa/inet.h>
int main() {
int sockfd;
struct ifconf ifconf;
struct ifreq ifreqs[20]; // 用于存储网络接口信息
char buf[128]; // 用于存储接口名字
sockfd = socket(AF_INET, SOCK_DGRAM, 0);
if (sockfd < 0) {
perror("socket");
exit(1);
}
ifconf.ifc_len = sizeof(ifreqs); // 设置ifconf的长度
ifconf.ifc_buf = (caddr_t) ifreqs; // 设置ifconf的缓冲区
if (ioctl(sockfd, SIOCGIFCONF, &ifconf) == -1) {
perror("ioctl");
exit(1);
}
struct ifreq* ifr = ifconf.ifc_req;
int numInterfaces = ifconf.ifc_len / sizeof(struct ifreq); // 获取网络接口数量
for (int i = 0; i < numInterfaces; i++) {
struct ifreq* item = &(ifr[i]);
ioctl(sockfd, SIOCGIFFLAGS, item); // 获取接口的标志位
if (item->ifr_flags & IFF_UP) { // 如果接口处于UP状态
if (item->ifr_flags & IFF_RUNNING) { // 如果接口处于Running状态
strncpy(buf, item->ifr_name, sizeof(buf)-1);
buf[sizeof(buf)-1] = '\0';
printf("WiFi接口名字: %s\n", buf);
}
}
}
close(sockfd);
return 0;
}
```
上述代码通过socket和ioctl函数调用来获取网络接口的信息,并检查接口的标志位来确定是否处于可用状态。最后,输出可用WiFi接口的名称。
请注意,代码中的错误检查和错误处理可能需要根据实际情况进行精细调整。此外,由于Linux系统中的网络接口命名可能因发行版而异,所以在其他Linux发行版上可能需要进行适当的修改。
### 回答3:
在Linux下,可以使用C语言编写代码来获取电脑可用的WiFi。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define MAX_BUFFER_SIZE 1024
int main() {
FILE *fp;
char buffer[MAX_BUFFER_SIZE];
// 执行iwlist命令获取WiFi列表信息
fp = popen("iwlist wlan0 scan", "r");
if (fp == NULL) {
printf("Error executing iwlist command\n");
return 1;
}
// 逐行读取输出结果并解析
while (fgets(buffer, sizeof(buffer), fp) != NULL) {
// 查找关键字来获取WiFi名称和信号强度
if (strstr(buffer, "ESSID:") != NULL) {
char *ssidStart = strchr(buffer, '\"');
if (ssidStart != NULL) {
char *ssidEnd = strchr(ssidStart + 1, '\"');
if (ssidEnd != NULL) {
*ssidEnd = '\0'; // 将双引号替换为字符串结束符
printf("WiFi名称:%s\n", ssidStart + 1);
}
}
}
if (strstr(buffer, "Signal level=") != NULL) {
char *signalStart = strstr(buffer, "Signal level=");
if (signalStart != NULL) {
char *signalEnd = strchr(signalStart + 1, ' ');
if (signalEnd != NULL) {
*signalEnd = '\0'; // 将空格替换为字符串结束符
printf("信号强度:%s\n", signalStart + 13);
}
}
}
}
// 关闭文件指针
pclose(fp);
return 0;
}
```
请注意,此示例代码使用了`iwlist`命令来扫描WiFi,并解析输出结果来获取WiFi名称和信号强度。需要确保已安装`iwlist`命令,并根据实际的无线网卡接口名称进行修改(如`wlan0`)。
相关推荐
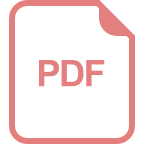















