用js编写一个宠物救助的网页
时间: 2024-06-08 16:05:50 浏览: 71
好的,下面是一个简单的宠物救助网页的示例,包括宠物列表、添加宠物和联系方式等功能。
HTML部分:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>宠物救助</title>
</head>
<body>
<h1>宠物救助</h1>
<h2>宠物列表</h2>
<ul id="pet-list">
<!-- 这里将会动态添加宠物列表 -->
</ul>
<h2>添加宠物</h2>
<form id="add-pet-form">
<label for="name">姓名:</label>
<input type="text" id="name" name="name"><br>
<label for="type">种类:</label>
<input type="text" id="type" name="type"><br>
<label for="age">年龄:</label>
<input type="text" id="age" name="age"><br>
<label for="description">描述:</label><br>
<textarea id="description" name="description"></textarea><br>
<button type="submit">添加宠物</button>
</form>
<h2>联系方式</h2>
<p>电话:1234567890</p>
<p>邮箱:rescue@pet.com</p>
<script src="app.js"></script>
</body>
</html>
```
JS部分:
```js
// 宠物数据
const pets = [];
// DOM元素
const petList = document.getElementById('pet-list');
const addPetForm = document.getElementById('add-pet-form');
// 从表单中获取宠物数据
function getPetData() {
const name = document.getElementById('name').value;
const type = document.getElementById('type').value;
const age = document.getElementById('age').value;
const description = document.getElementById('description').value;
return { name, type, age, description };
}
// 渲染宠物列表
function renderPetList() {
petList.innerHTML = '';
pets.forEach(pet => {
const li = document.createElement('li');
li.innerHTML = `
<h3>${pet.name} (${pet.type})</h3>
<p>年龄:${pet.age}</p>
<p>描述:${pet.description}</p>
`;
petList.appendChild(li);
});
}
// 添加宠物
function addPet(event) {
event.preventDefault();
const pet = getPetData();
pets.push(pet);
renderPetList();
addPetForm.reset();
}
// 绑定事件
addPetForm.addEventListener('submit', addPet);
// 初始渲染
renderPetList();
```
这是一个非常简单的示例,仅供参考。您可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
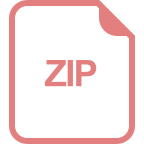
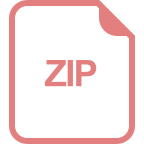
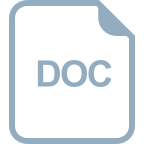
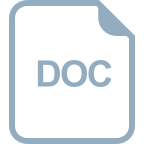





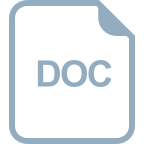
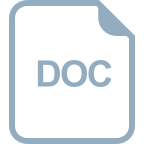
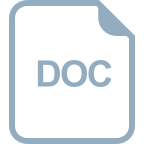
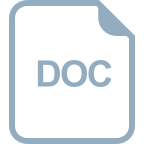
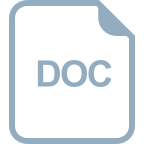
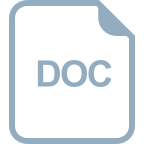


