用 51 单片机,DS18B20 数字温度传感器,三合一共阳数码管,数码管建议采用专用芯片 TM1637 驱动,五个功能按键,一 个蜂鸣器
时间: 2023-10-03 12:05:24 浏览: 157
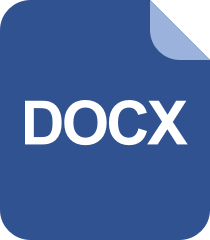
c51单片机数码管显示温度
你需要一个51单片机来控制这个系统,可以连接DS18B20数字温度传感器来读取温度数据,并使用TM1637芯片来驱动三合一共阳数码管显示温度。五个功能按键可以用来控制系统的不同功能,例如设置温度报警阈值等。蜂鸣器可以用来发出警报声音。以下是基本的硬件连接和代码示例:
硬件连接:
DS18B20传感器连接单片机的P3口;
TM1637芯片连接单片机的P1口和P2口;
五个功能按键分别连接单片机的P4-P8口;
蜂鸣器连接单片机的P0口。
代码示例:
```c
#include <reg52.h>
#define uchar unsigned char
#define uint unsigned int
sbit DSPORT = P3^7; // DS18B20传感器数据线连接P3.7口
sbit DSWRITE = P3^6; // DS18B20传感器写数据线连接P3.6口
sbit DSREAD = P3^5; // DS18B20传感器读数据线连接P3.5口
sbit DSRST = P3^4; // DS18B20传感器复位线连接P3.4口
sbit CLK = P1^0; // TM1637时钟线连接P1.0口
sbit DIO = P1^1; // TM1637数据线连接P1.1口
sbit KEY1 = P4^0; // 第一个功能按键连接P4.0口
sbit KEY2 = P5^0; // 第二个功能按键连接P5.0口
sbit KEY3 = P6^0; // 第三个功能按键连接P6.0口
sbit KEY4 = P7^0; // 第四个功能按键连接P7.0口
sbit KEY5 = P8^0; // 第五个功能按键连接P8.0口
sbit BEEP = P0^0; // 蜂鸣器连接P0.0口
uchar code digit_table[] = { // 数码管显示字母和数字的编码表
0x3f, 0x06, 0x5b, 0x4f, 0x66, 0x6d, 0x7d, 0x07, 0x7f, 0x6f, // 0-9
0x77, 0x7c, 0x39, 0x5e, 0x79, 0x71, 0x3d, 0x76, 0x06, 0x1e, // A-J
0x0f, 0x38, 0x15, 0x54, 0x5c, 0x73, 0x67, 0x50, 0x6d, 0x78, // K-T
0x3e, 0x1c, 0x7e, 0x6e, 0x5b, 0x0c, 0x7f, 0x39, 0x74, 0x00 // U-Z, -
};
// 延时函数
void delay(uint t) {
while(t--);
}
// DS18B20传感器初始化
uchar ds18b20_init() {
uchar init_flag;
DSPORT = 1;
DSWRITE = 1;
DSREAD = 1;
DSRST = 1;
delay(1);
DSRST = 0;
delay(500);
DSRST = 1;
delay(1);
init_flag = DSREAD;
delay(500);
return init_flag;
}
// DS18B20传感器写一个字节
void ds18b20_writebyte(uchar dat) {
uchar i;
for(i=0; i<8; i++) {
DSWRITE = 0;
DSPORT = dat & 0x01;
delay(5);
DSWRITE = 1;
dat >>= 1;
}
}
// DS18B20传感器读一个字节
uchar ds18b20_readbyte() {
uchar i, dat = 0;
for(i=0; i<8; i++) {
DSWRITE = 0;
DSPORT = 1;
delay(2);
dat >>= 1;
if(DSREAD) dat |= 0x80;
delay(5);
DSWRITE = 1;
}
return dat;
}
// 读取DS18B20传感器温度数据
void ds18b20_readtemp(uchar *temp) {
uchar TL, TH;
ds18b20_init();
ds18b20_writebyte(0xcc);
ds18b20_writebyte(0x44);
delay(100); // 延时等待转换完成
ds18b20_init();
ds18b20_writebyte(0xcc);
ds18b20_writebyte(0xbe);
TL = ds18b20_readbyte();
TH = ds18b20_readbyte();
*temp = (TH << 4) | (TL >> 4); // 取整数部分
}
// TM1637芯片发送一个字节数据
void tm1637_sendbyte(uchar dat) {
uchar i;
CLK = 1;
for(i=0; i<8; i++) {
DIO = dat & 0x01;
dat >>= 1;
CLK = 0;
delay(1);
CLK = 1;
}
}
// TM1637芯片显示一个数码管
void tm1637_display(uchar addr, uchar dat) {
tm1637_sendbyte(0x44);
CLK = 0;
DIO = 0;
CLK = 1;
tm1637_sendbyte(0xc0 | addr);
tm1637_sendbyte(digit_table[dat]);
tm1637_sendbyte(0x88);
}
// 主函数
void main() {
uchar temp;
tm1637_display(0, 0);
tm1637_display(1, 0);
tm1637_display(2, 0);
tm1637_display(3, 0);
while(1) {
ds18b20_readtemp(&temp);
tm1637_display(0, temp / 100);
tm1637_display(1, temp % 100 / 10);
tm1637_display(2, temp % 10);
delay(500);
if(KEY1 == 0) { // 第一个功能按键被按下
BEEP = 1; // 发出警报声音
delay(1000);
BEEP = 0;
}
if(KEY2 == 0) { // 第二个功能按键被按下
// 设置温度报警阈值等
}
// 其他功能按键的处理
}
}
```
阅读全文
相关推荐
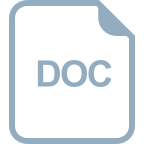
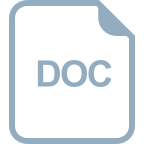
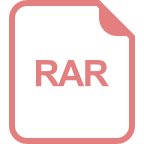

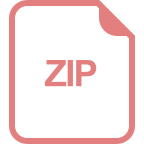
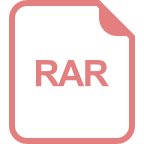
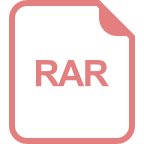
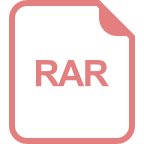
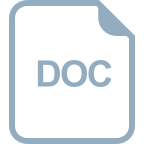
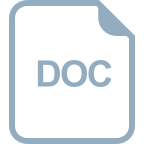
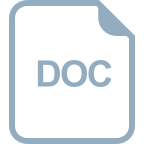


