Python代码实现A星寻路算法
时间: 2023-08-31 09:19:03 浏览: 325
### 回答1:
Python代码实现A星寻路算法可以使用以下代码:def astar(start, goal):
closedset = set()
openset = set([start])
came_from = {}
gscore = {start: 0}
fscore = {start: heuristic_cost_estimate(start, goal)}
while openset:
current = min(openset, key=fscore.get)
if current == goal:
return reconstruct_path(came_from, current)
openset.remove(current)
closedset.add(current)
for neighbor in get_neighbors(current):
if neighbor in closedset:
continue
tentative_gscore = gscore[current] + dist_between(current, neighbor)
if neighbor not in openset:
openset.add(neighbor)
elif tentative_gscore >= gscore[neighbor]:
continue
came_from[neighbor] = current
gscore[neighbor] = tentative_gscore
fscore[neighbor] = gscore[neighbor] + heuristic_cost_estimate(neighbor, goal)
return False
### 回答2:
A星寻路算法是一种常用的路径规划算法,可以在给定的地图中找到最短路径。下面是用Python实现A星寻路算法的代码示例:
```python
import heapq
# 定义节点类
class Node:
def __init__(self, row, col, g, h):
self.row = row
self.col = col
self.g = g
self.h = h
def __lt__(self, other):
return self.g + self.h < other.g + other.h
# 计算启发式代价(h值)
def heuristic(row, col, target_row, target_col):
return abs(target_row - row) + abs(target_col - col)
# A星寻路算法
def astar_search(start, target, grid):
directions = [(-1, 0), (1, 0), (0, -1), (0, 1)] # 上下左右四个方向
rows, cols = len(grid), len(grid[0])
visited = set() # 已访问的节点集合
pq = [] # 优先队列,用来选择下一个节点
heapq.heappush(pq, start)
while pq:
curr_node = heapq.heappop(pq)
row, col = curr_node.row, curr_node.col
visited.add((row, col))
if row == target.row and col == target.col:
return curr_node.g
for d in directions:
new_row, new_col = row + d[0], col + d[1]
if 0 <= new_row < rows and 0 <= new_col < cols and grid[new_row][new_col] != 1:
new_g = curr_node.g + 1
new_h = heuristic(new_row, new_col, target.row, target.col)
new_node = Node(new_row, new_col, new_g, new_h)
if (new_row, new_col) not in visited:
heapq.heappush(pq, new_node)
return -1
# 示例
grid = [[0, 0, 0], [1, 1, 0], [0, 0, 0]]
start = Node(0, 0, 0, 0)
target = Node(2, 2, 0, 0)
result = astar_search(start, target, grid)
print(result)
```
以上代码实现了A星寻路算法,并可用于寻找给定地图中起点到目标点的最短路径。其中,`grid`是二维列表表示地图,在地图中,0表示可通过的节点,1表示不可通过的节点。`start`表示起点,`target`表示目标点。通过调用`astar_search`函数,将起点、目标点和地图作为参数进行传递,即可得到最短路径的长度。
### 回答3:
A星寻路算法是一种常用于寻找最短路径的算法,广泛应用于游戏开发、路径规划等领域。下面是使用Python实现A星寻路算法的代码:
```python
# 定义地图和起终点
map = [
[0, 0, 0, 0, 0, 0, 0],
[0, 1, 1, 0, 0, 0, 0],
[0, 0, 1, 0, 0, 0, 0],
[0, 0, 1, 1, 1, 1, 0],
[0, 0, 0, 0, 0, 1, 0],
[0, 0, 0, 0, 0, 1, 0],
[0, 0, 0, 0, 0, 0, 0]
]
start = (1, 1) # 起点坐标
goal = (6, 5) # 终点坐标
# 定义辅助函数
def heuristic(pos1, pos2):
# 估算两点之间的距离(启发函数)
x1, y1 = pos1
x2, y2 = pos2
return abs(x1 - x2) + abs(y1 - y2)
def get_neighbors(pos):
# 获取当前位置的邻近位置
x, y = pos
neighbors = []
for dx in [-1, 0, 1]:
for dy in [-1, 0, 1]:
if dx == dy == 0: # 排除当前位置
continue
new_x, new_y = x + dx, y + dy
if 0 <= new_x < len(map) and 0 <= new_y < len(map[0]) and map[new_x][new_y] == 0:
neighbors.append((new_x, new_y))
return neighbors
# 定义A星算法函数
def astar_search(start, goal):
# 初始化起点和终点
open_list = [start] # 待探索的节点
came_from = {} # 记录路径中每个节点的上一个节点
g_score = {start: 0} # 起点到每个节点的实际代价
f_score = {start: heuristic(start, goal)} # 起点到每个节点的估算代价(f_score = g_score + h_score)
while open_list:
current = min(open_list, key=lambda x: f_score[x]) # 获取f_score最小的节点
if current == goal: # 已到达终点
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
open_list.remove(current)
for neighbor in get_neighbors(current):
g_temp = g_score[current] + 1 # 节点到邻近节点的代价为1
if neighbor not in g_score or g_temp < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = g_temp
f_score[neighbor] = g_temp + heuristic(neighbor, goal)
if neighbor not in open_list:
open_list.append(neighbor)
return [] # 未找到路径
# 在地图上运行A星算法
path = astar_search(start, goal)
print("路径: ", path)
```
该代码首先定义了一个地图,使用0表示可行走的区域,使用1表示障碍物。将起点和终点设定好后,通过`heuristic`函数计算两点间的估算距离。`get_neighbors`函数用于获取当前位置的邻近位置。
`astar_search`函数是实现A星算法的核心部分。其中,`open_list`用于存放待探索的节点,`came_from`用于记录路径中每个节点的上一个节点,`g_score`用于记录起点到每个节点的实际代价,`f_score`用于记录起点到每个节点的估算代价。算法使用一个循环不断探索下一个最有可能的节点,直到找到终点或无法找到路径为止。
最后,在地图上运行A星算法,并输出结果路径。
阅读全文
相关推荐
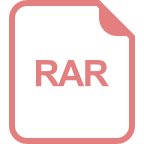
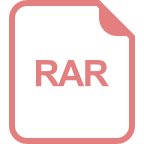
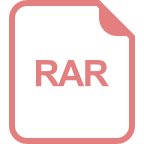
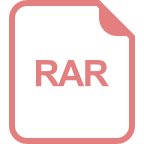
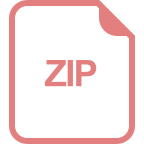
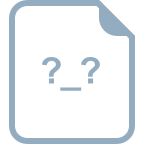
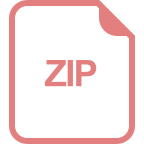
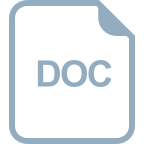
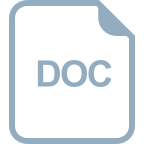
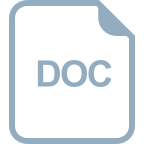
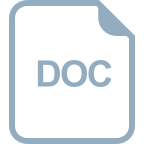





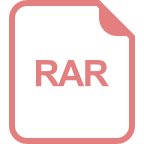
