可以在线程中销毁自身么
时间: 2023-12-30 13:06:30 浏览: 60
在 Python 中,线程对象并不支持自我销毁。如果在线程中需要结束自身,可以使用一些技巧来实现。以下是两种常见的方法:
1. 使用标志变量:在线程中使用一个标志变量来表示线程是否需要退出,当需要退出时,设置标志变量并等待线程执行完成后退出。例如:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
super().__init__()
self._stop_event = threading.Event()
def run(self):
while not self._stop_event.is_set():
print("Running...")
time.sleep(1)
print("Stopped")
def stop(self):
self._stop_event.set()
t = MyThread()
t.start()
# 在需要的时候停止线程
time.sleep(5)
t.stop()
t.join()
```
在上面的代码中,我们创建了一个 `MyThread` 类,并在其中定义了一个标志变量 `_stop_event`,用于表示线程是否需要退出。在线程的 `run()` 方法中,我们使用循环来执行任务,并在每次循环中检查 `_stop_event` 是否被设置为 `True`,如果被设置为 `True`,则退出循环并打印 "Stopped"。在主线程中,我们等待 5 秒钟后调用 `t.stop()` 方法来设置 `_stop_event`,然后使用 `t.join()` 等待线程 `t` 执行完成并销毁线程对象。
2. 使用异常:在线程中抛出一个异常来结束线程,并在主线程中捕获异常来销毁线程对象。例如:
```python
import threading
import time
class MyThread(threading.Thread):
def run(self):
try:
while True:
print("Running...")
time.sleep(1)
except KeyboardInterrupt:
print("Stopped")
t = MyThread()
t.start()
# 在需要的时候停止线程
time.sleep(5)
t._stop()
t.join()
```
在上面的代码中,我们在线程的 `run()` 方法中使用一个无限循环来执行任务,并在循环内部捕获 `KeyboardInterrupt` 异常来结束线程。在主线程中,我们等待 5 秒钟后调用 `t._stop()` 方法来抛出 `KeyboardInterrupt` 异常,然后使用 `t.join()` 等待线程 `t` 执行完成并销毁线程对象。
需要注意的是,第二种方法可能会在某些情况下导致线程无法正常退出,因为线程可能会在某个不受控制的时刻抛出 `KeyboardInterrupt` 异常。因此,第一种方法更加安全和可靠。
阅读全文
相关推荐
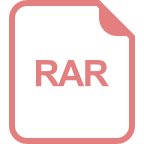
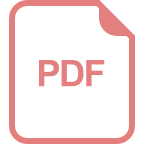
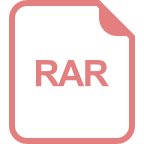
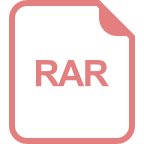
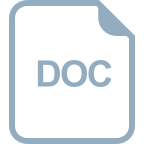



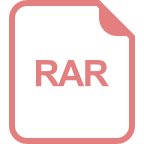
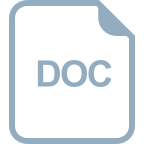
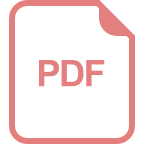
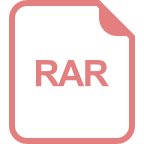
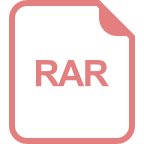
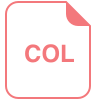
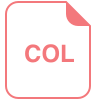


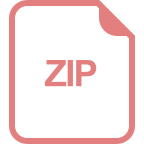